布局和页面跳转
页面布局
布局其实就是容器组件
解决怎么摆放普通组件
的问题。
容器组件
本质上是一颗树型结构。
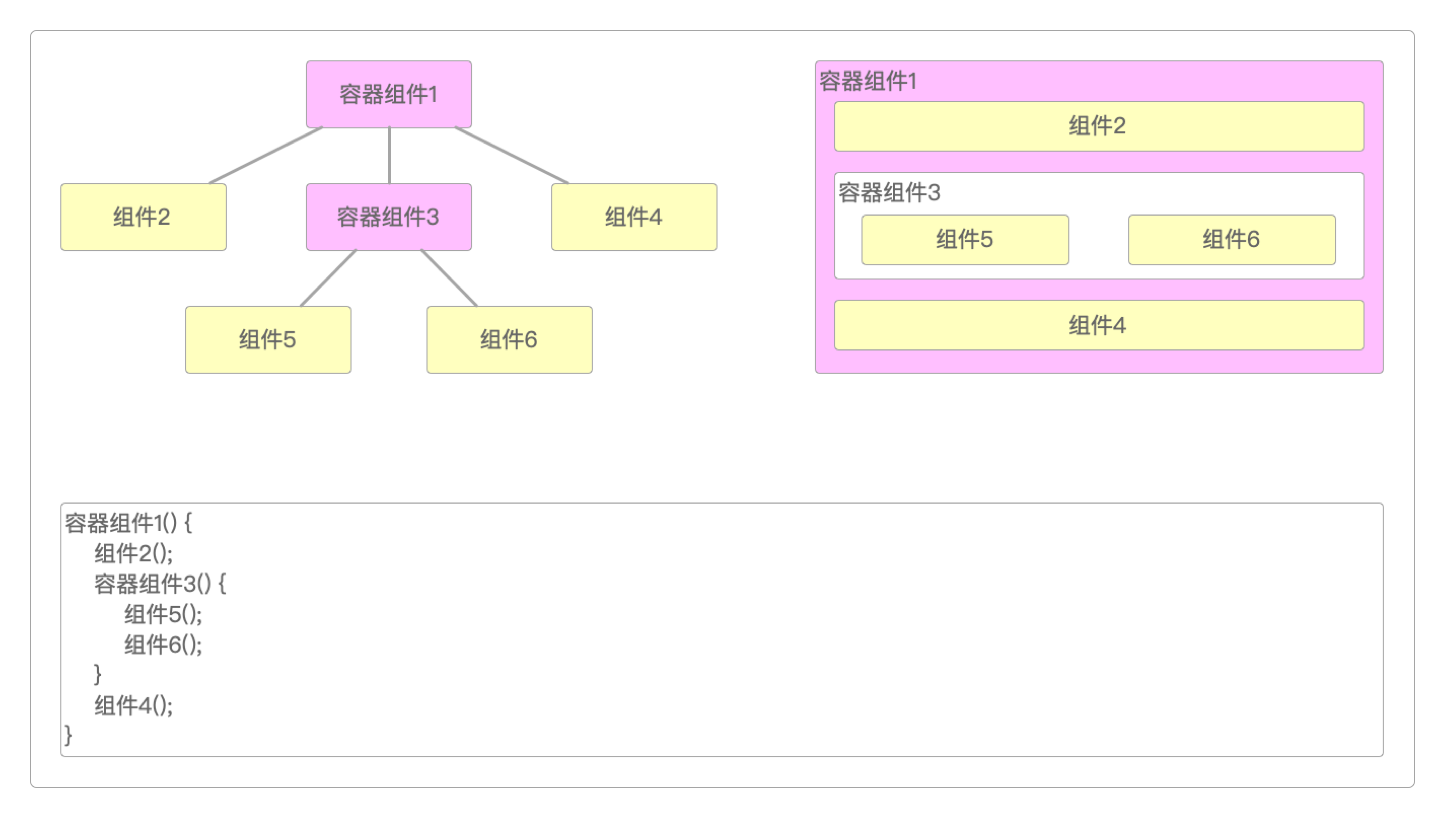
在开发中通过build()
构建的整个界面也是一棵树,它和组件都可以通过参数进行初始化。
@Component
struct 自定义组件名 {
// 成员变量和方法
build() {
容器组件1() {
组件2()...属性设置;
容器组件3([参数]) {
组件5()...属性设置;
组件6()...属性设置;
}
组件4()...属性设置;
}...属性设置;
}
}
HarmonyOS官方列举了十大布局,但开发中常见的布局有以下八种。
一、线性布局(LinearLayout)
线性布局(LinearLayout):通过线性容器Row(行)
和Column(列)
构建,子元素在线性方向上(水平或垂直)依次排列,是其他布局的基础。
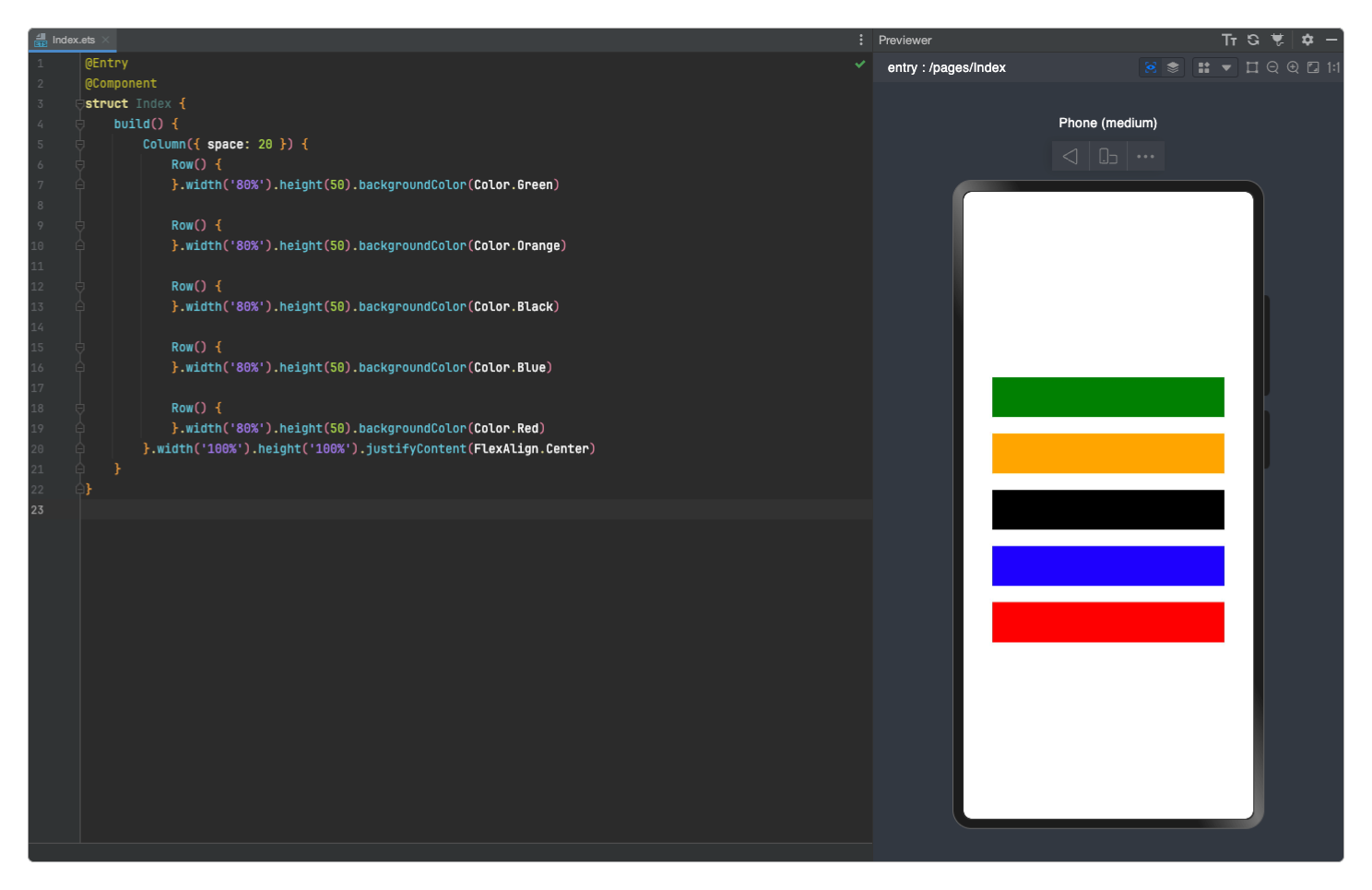
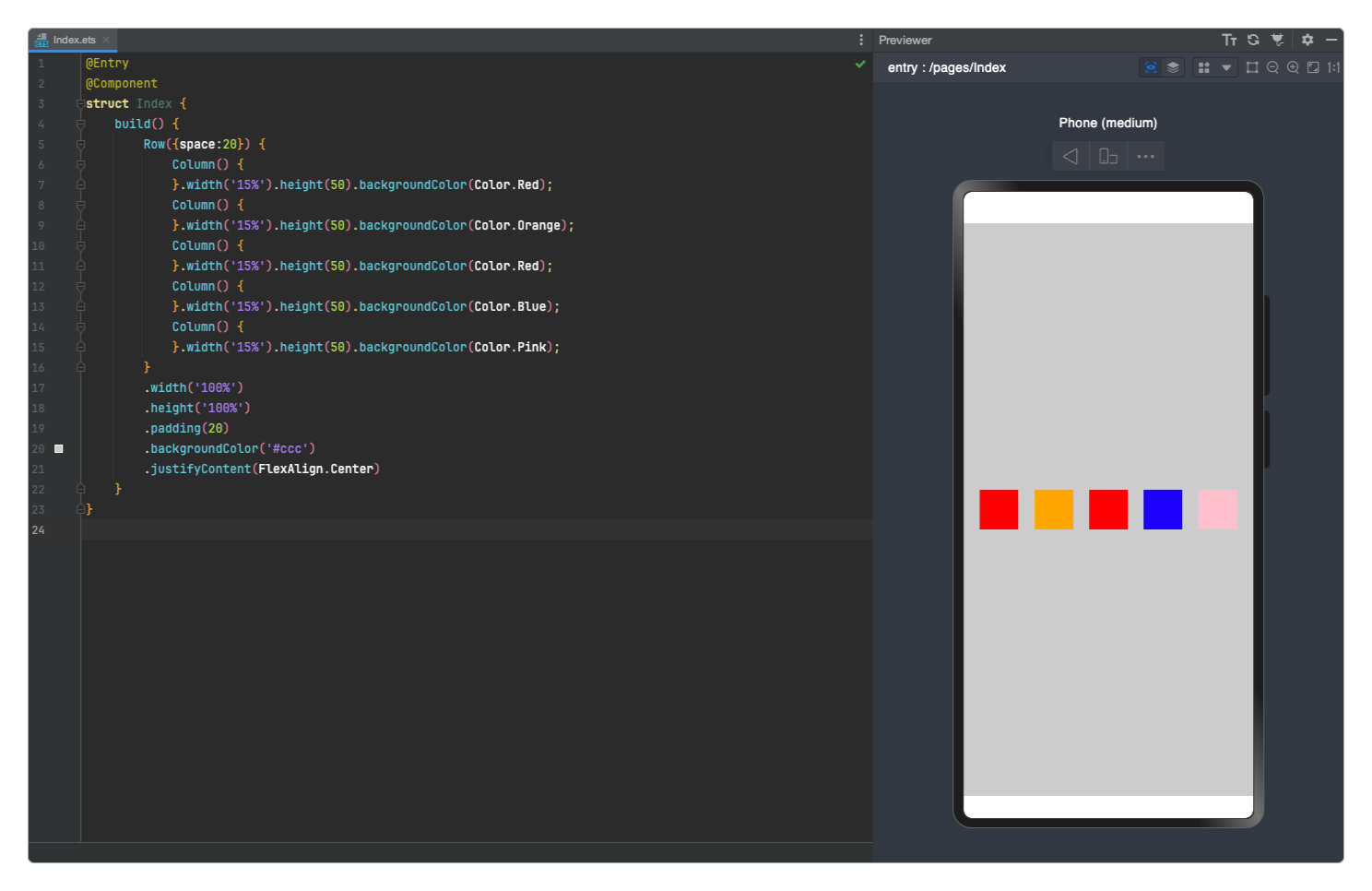
二、弹性布局(Flex)
弹性布局(Flex):当容器中子元素尺寸的总和大于容器尺寸时,子元素尺寸会自动挤压以适应容器大小。
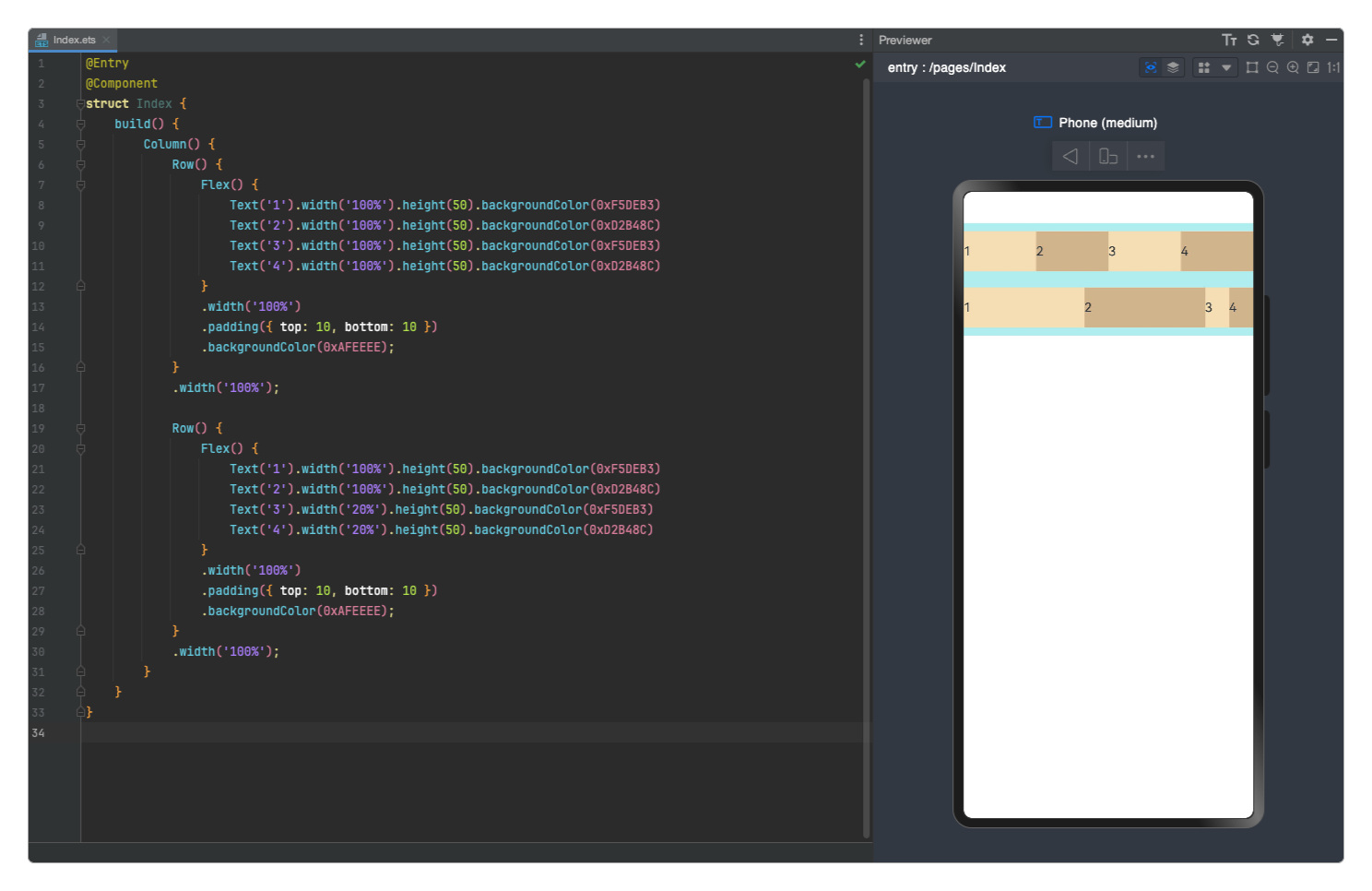
三、层叠布局(Stack)
层叠布局(Stack):将子元素沿垂直于屏幕的方向堆叠在一起,类似于图层的叠加。
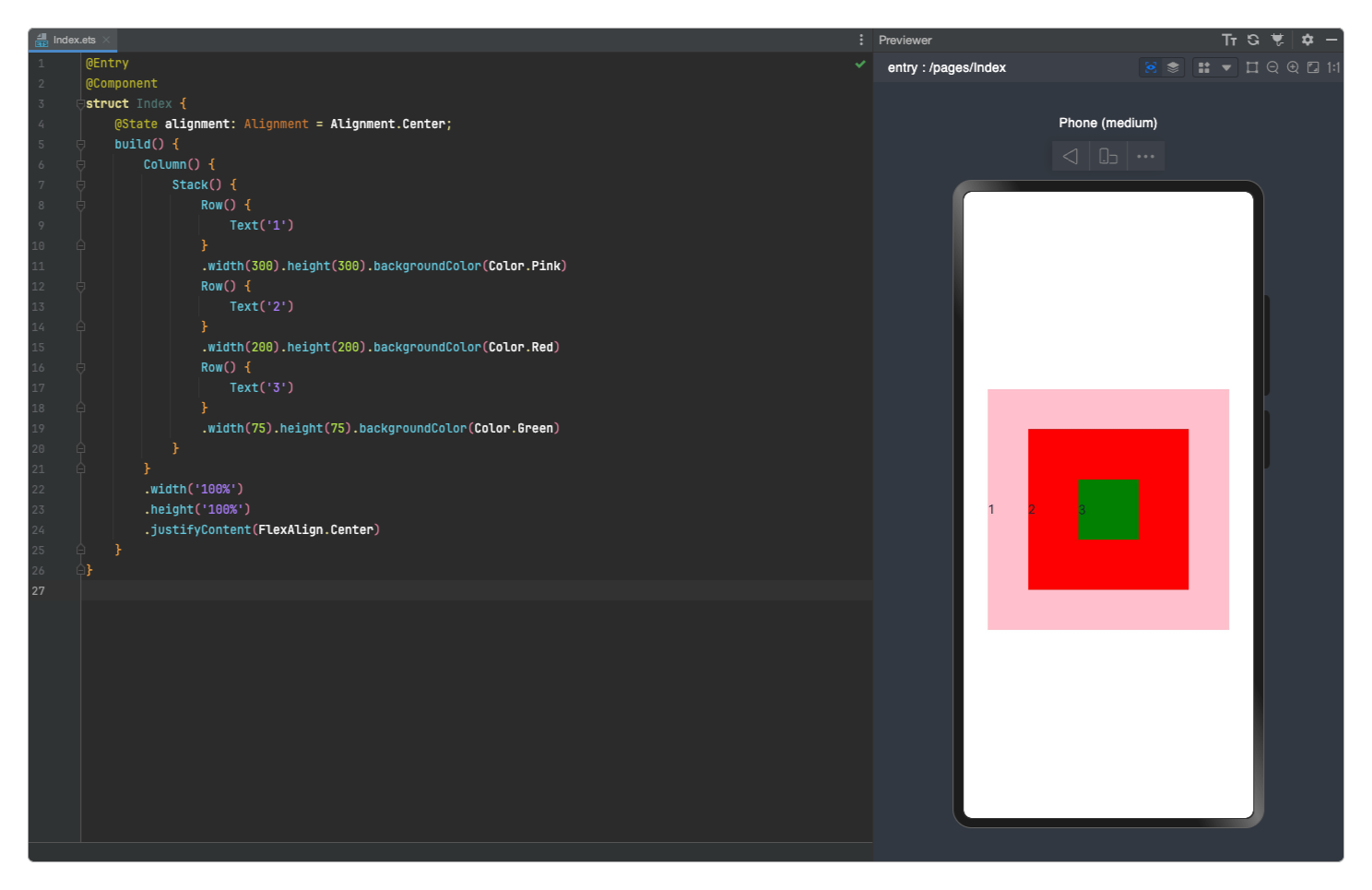
四、列表布局(List)
列表布局(List):使用列表可以轻松高效地显示结构化、可滚动的列表信息。
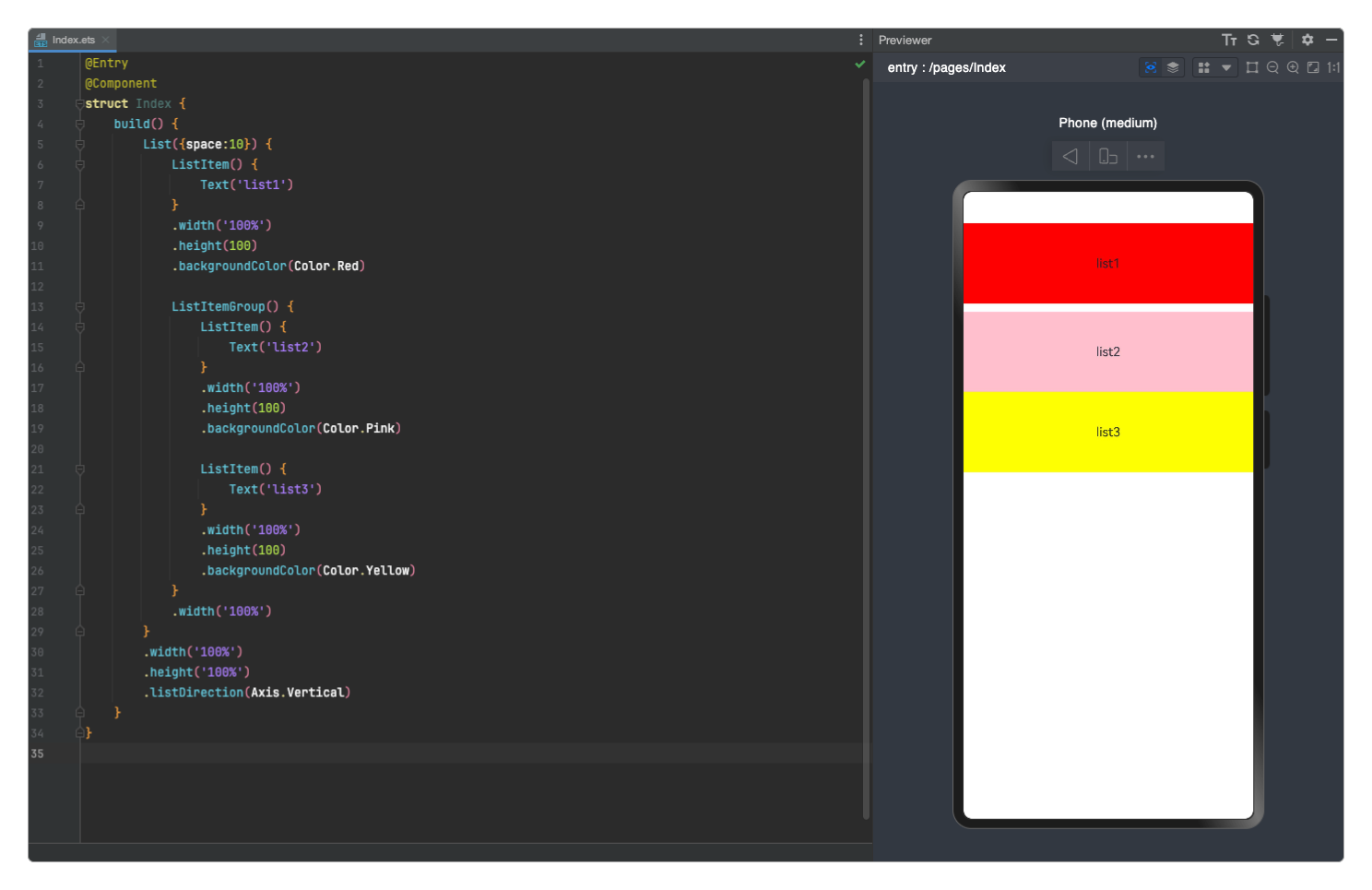
五、网格布局(Grid)
网格布局(Grid):通过将页面划分为行和列组成的网格,使得子组件可以在这个二维网格中自由定位。
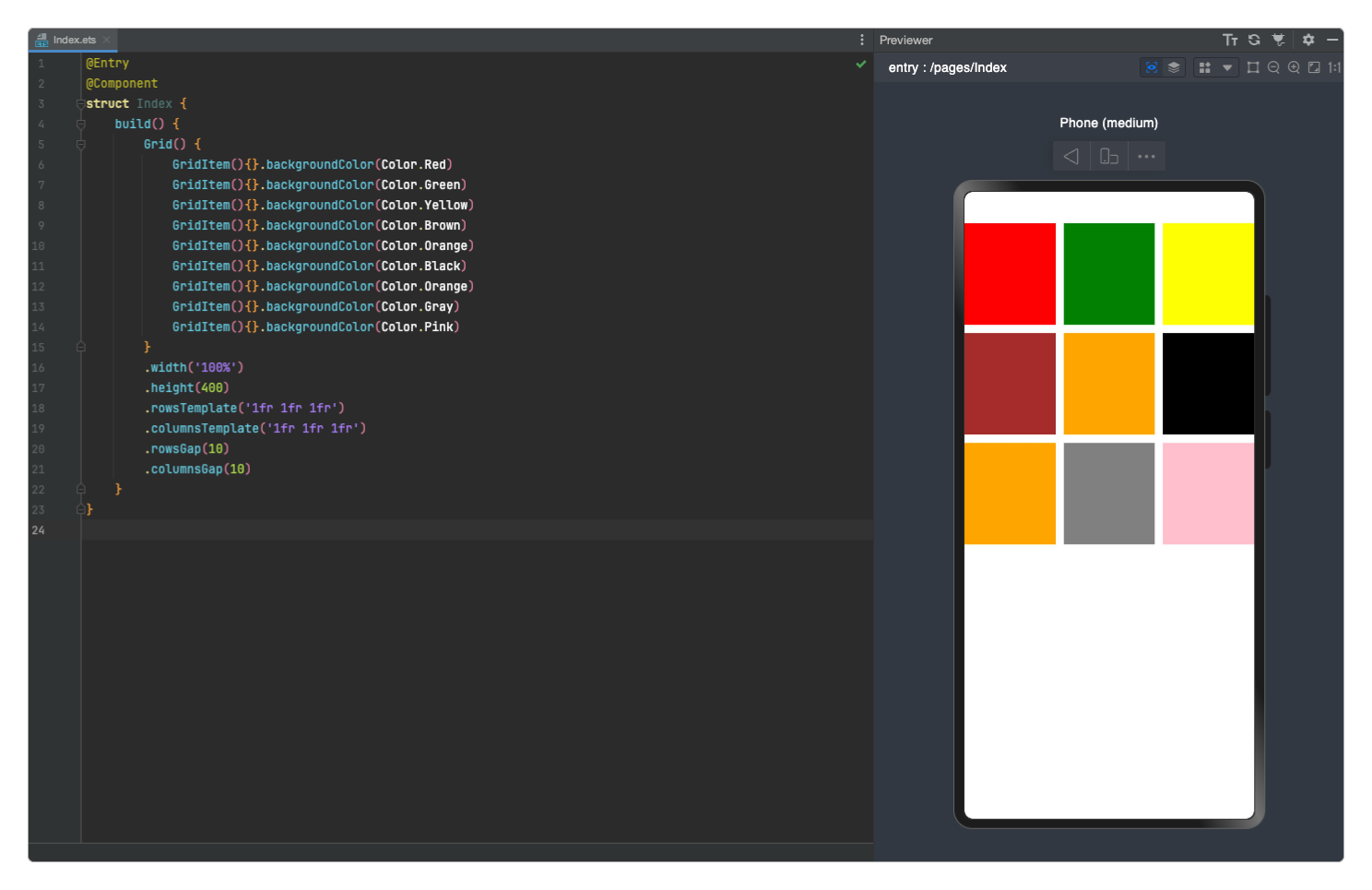
六、相对布局(RelativeContainer)
相对布局(RelativeContainer):支持容器内部的子元素设置相对位置关系,适用于界面复杂的情况。
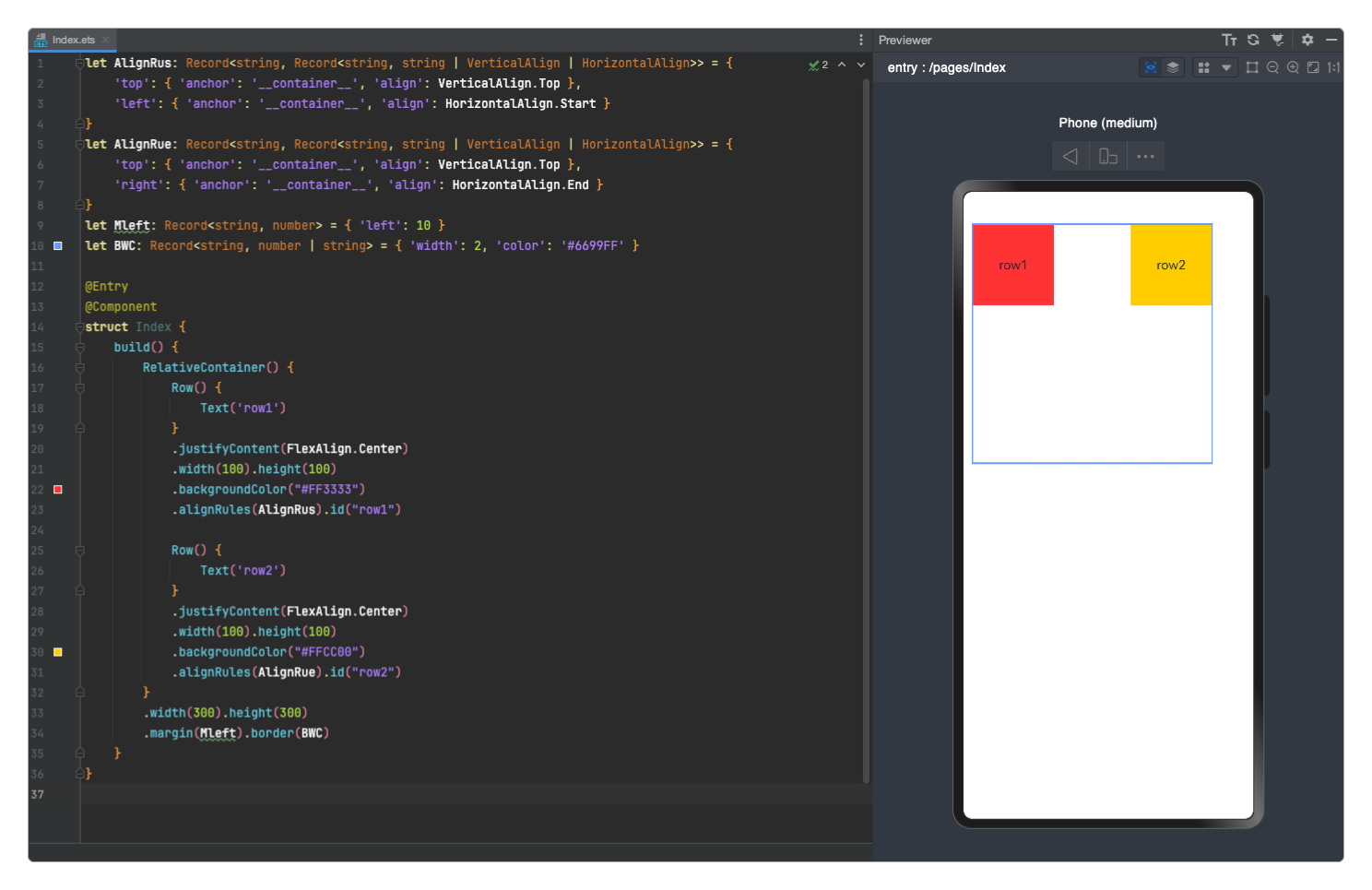
七、选项卡布局(Tabs)
选项卡布局(Tabs):通过对页面内容进行分类,在一个页面内快速实现视图内容的切换。
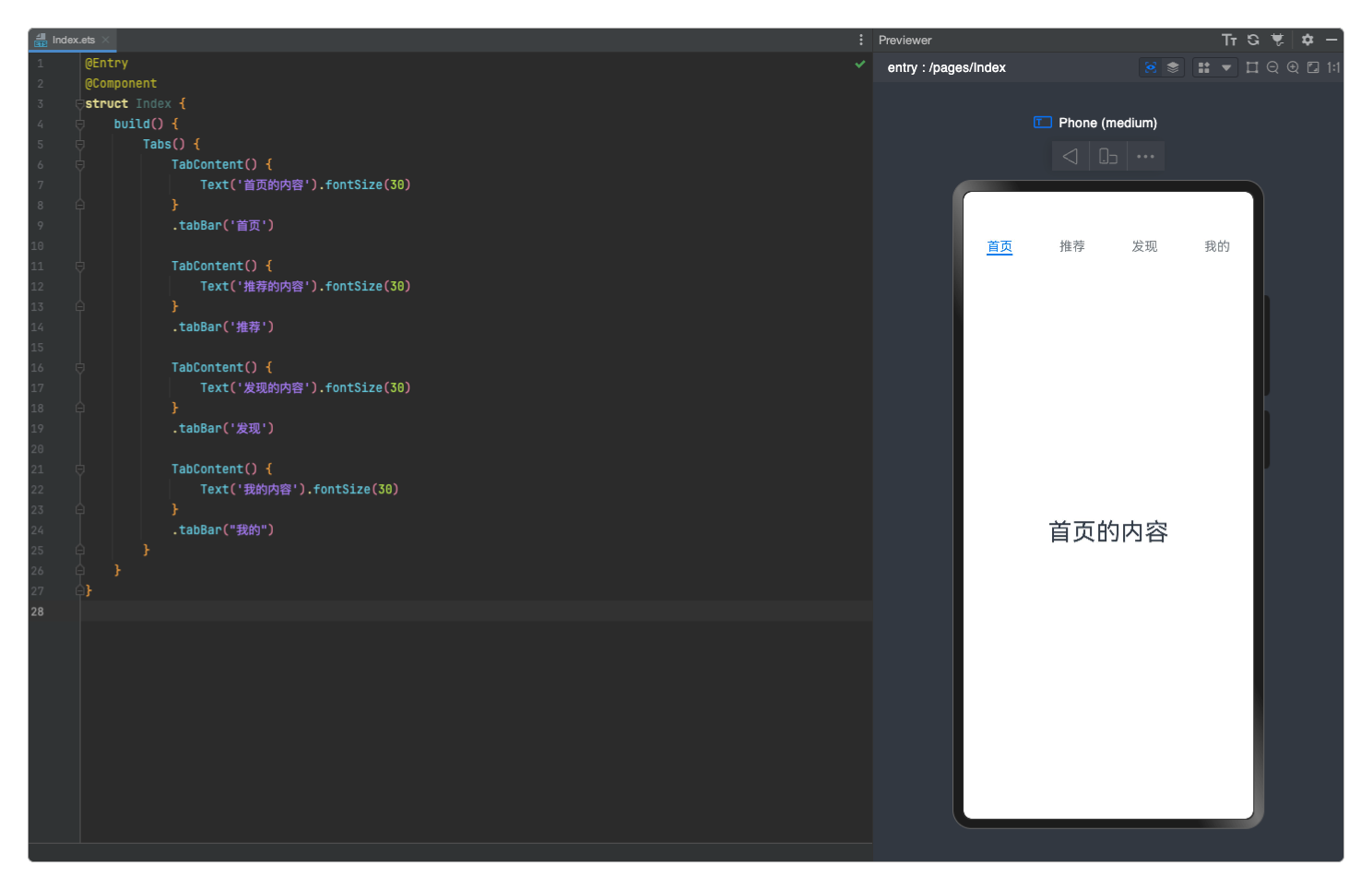
八、轮播布局(Swiper)
轮播布局(Swiper):Swiper组件提供滑动轮播显示的能力。Swiper本身是一个容器组件,当设置了多个子组件后,可以对这些子组件进行轮播显示。
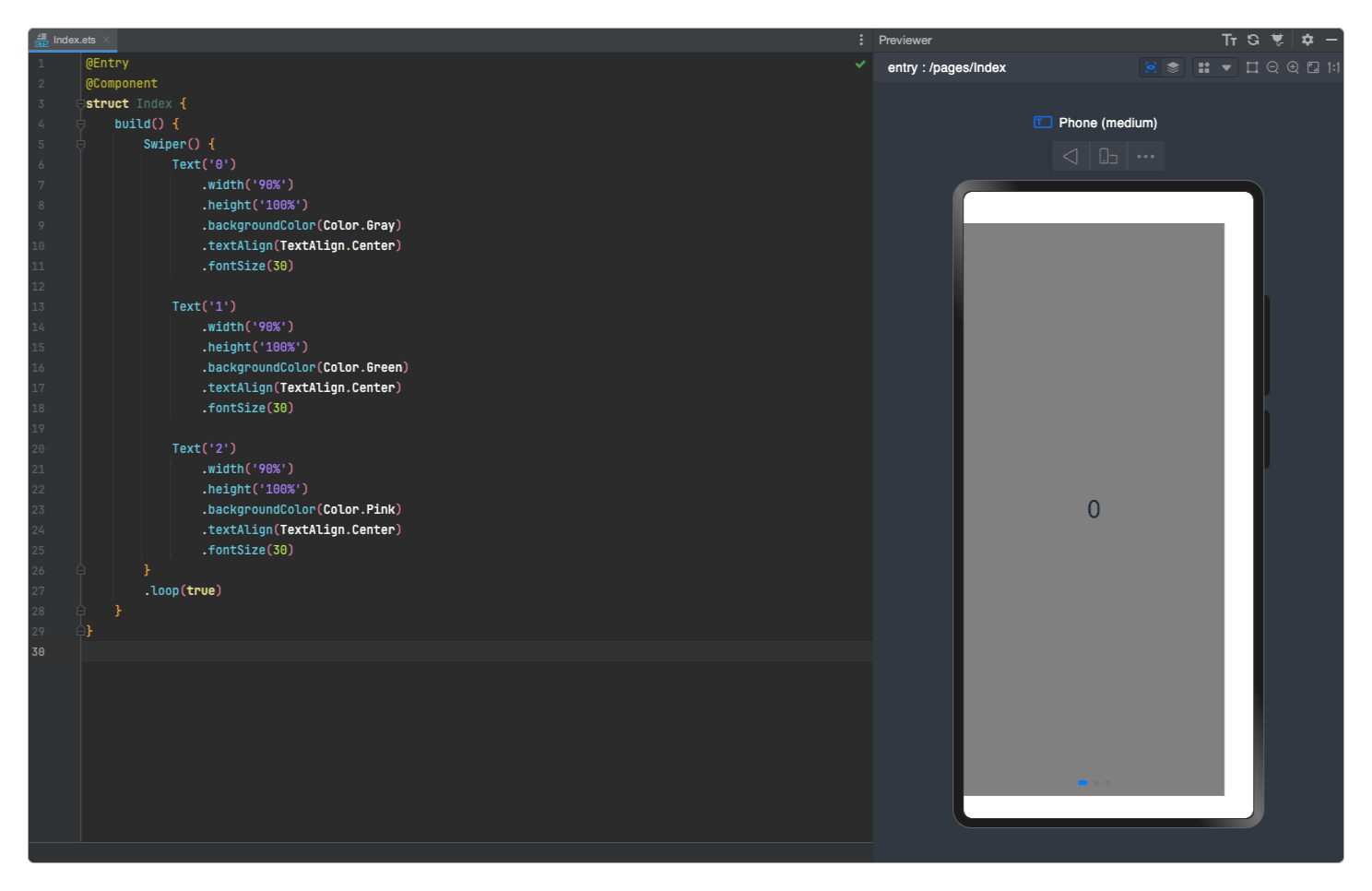
页面跳转
在ArkUI中,一个应用可以包含多个Ability(能力)
,每个Ability
又可以包含若干个Page(页面)
,一个Page
对应一个.ets
文件。
这里的跳转指的是在同一个Ability
中多个不同Page
之间的跳转。ArkUI提供了两种实现方式。
使用导航组件
Navigator
实现,它有三种跳转方式。使用
路由接口API
实现,也对应地有三种方式。router.pushUrl()
。router.replaceUrl()
。router.back()
。
Index.ets
首页代码。
// 导入路由模块
import router from '@ohos.router'
@Entry
@Component
struct Index {
message: string = '主页面'
@State count: number = 1
build() {
Row() {
Column() {
Text(this.message)
.fontSize(50)
.fontWeight(FontWeight.Bold)
Row() {
Text("请输入数量:").fontSize(28)
// 计数器组件
Counter() {
Text(this.count.toString()).fontSize(25)
}.margin(5).height(32)
.onInc(() => {
this.count++
})
.onDec(() => {
this.count--
})
}.padding(5).backgroundColor(0xEEEEEE)
.justifyContent(FlexAlign.SpaceEvenly)
.margin(20)
// 导航组件
Navigator({ target: 'pages/Other' }) {
Column() {
Text('导航跳转到Other')
.fontSize(30).backgroundColor(0xEEFFEE)
.fontWeight(FontWeight.Bold)
}.width("100%").backgroundColor(0xFFEEEE)
.padding(5)
}.margin(20)
// 携带参数
.params({ data: this.count })
// 路由组件
Button('路由跳转到Other')
.fontSize(30)
.fontWeight(FontWeight.Bold)
.width("90%")
.margin(20)
.onClick(() => {
router.pushUrl({
url: 'pages/Other',
// 携带参数
params: { data: this.count }
})
})
}
.width('100%')
}
.height('100%')
}
}
Other.ets
页面代码。
import router from '@ohos.router'
@Entry
@Component
struct Other {
message: string = 'Other页面'
// 获取参数对象
@State params: object = router.getParams()
build() {
Row() {
Column() {
Text(this.message).fontSize(50).fontWeight(FontWeight.Bold);
// 通过下标i形式使用参数中的数据
if (this.params != undefined) {
Text("传递的数值:" + this.params['data']).fontSize(30);
} else {
Text("没有获得传递参数").fontSize(30).fontColor(0xff0000);
}
Navigator({ target: 'pages/Other' }) {
Column() {
Text('跳转到Other页面')
.fontSize(30).backgroundColor(0xEEFFEE)
.fontWeight(FontWeight.Bold);
}.width("100%")
.backgroundColor(0xFFEEEE).padding(15)
}.margin(20).active(false);
Navigator({ target: 'pages/Another' }) {
Column() {
Text('跳转到Another页面')
.fontSize(30).backgroundColor(0xEEFFEE)
.fontWeight(FontWeight.Bold);
}.width("100%").backgroundColor(0xFFEEEE)
.padding(15)
}.margin(20).active(false)
}
.width('100%')
}
.height('100%')
}
}
Another.ets
页面代码。
import router from '@ohos.router';
@Entry
@Component
struct Goto {
message: string = 'Another页面'
build() {
Row() {
Column() {
Text(this.message).fontSize(50).fontWeight(FontWeight.Bold);
Button('router返回')
.fontSize(30)
.fontWeight(FontWeight.Bold)
.width("90%")
.margin(20)
.onClick(() => {
// 无参数
router.back();
});
Navigator({
target: 'pages/Other',
type: NavigationType.Back
}) {
Column() {
Text('Navigator返回').fontSize(30).backgroundColor(0xEEFFEE).fontWeight(FontWeight.Bold);
}.width("100%").backgroundColor(0xFFEEEE)
.padding(15)
}.margin(20);
Navigator({
target: 'pages/Index',
type: NavigationType.Back
}) {
Column() {
Text('Navigator到Index').fontSize(30).backgroundColor(0xEEFFEE).fontWeight(FontWeight.Bold);
}.width("100%").backgroundColor(0xFFEEEE)
.padding(15)
}.margin(20);
}
.width('100%')
}
.height('100%')
}
}
感谢支持
更多内容,请移步《超级个体》。