异步的承诺
原创大约 2 分钟
泡茶的逻辑
在讲到并行时,有些资料中会提到关于泡茶的例子——也就是有两种泡茶方式。
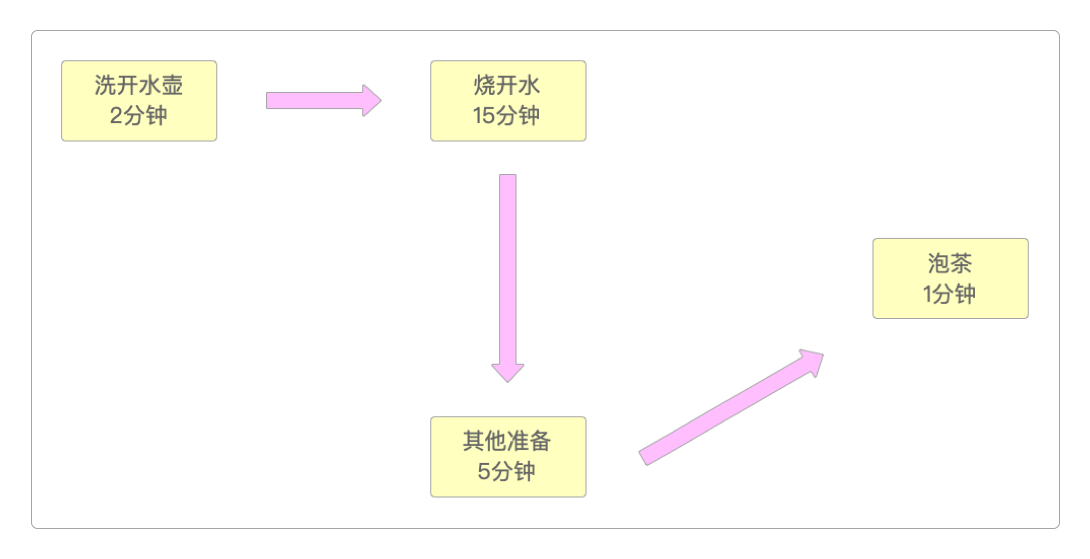
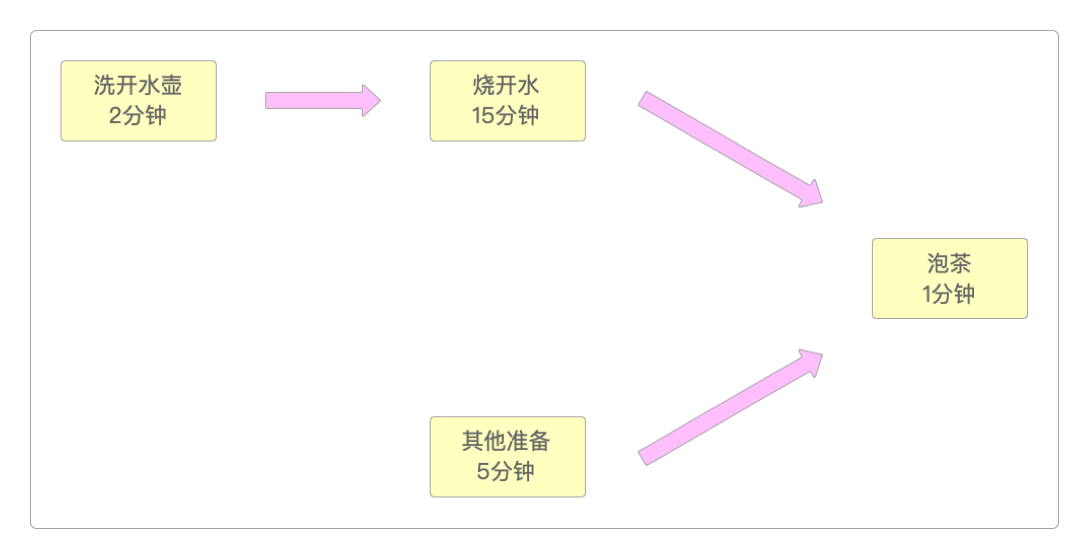
可以看到,这两种方式消耗的时间是不同的。
完全可以在烧开水的同时去做其他的准备工作,例如,洗茶杯、准备茶叶等,把烧开水和其他准备事项并行起来做,可以大大节约总的耗时时间。
先用代码来展示第一种串行的泡茶方式
。
/**
* 烧开水
*
*/
public class BoilWater {
private boolean status = false;
public boolean isStatus() {
return status;
}
public void setStatus(boolean status) {
this.status = status;
}
}
/**
* 其他准备工作
*
*/
public class OtherPreparation {
private boolean status = false;
public boolean isStatus() {
return status;
}
public void setStatus(boolean status) {
this.status = status;
}
}
/**
* 串行的泡茶步骤
*
*/
public class SerialMakeTea {
public static void main(String[] args) throws InterruptedException {
long start = System.currentTimeMillis();
Thread t1 = new Thread(() -> {
System.out.println("烧水开始:需要5分钟");
try {
BoilWater bw = new BoilWater();
bw.setStatus(true);
TimeUnit.SECONDS.sleep(5);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("烧水结束");
});
t1.start();
t1.join();
Thread t2 = new Thread(() -> {
System.out.println("其他准备开始:需要2分钟");
try {
OtherPreparation tc = new OtherPreparation();
tc.setStatus(true);
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
throw new RuntimeException(e);
}
System.out.println("其他准备结束");
});
t2.start();
t2.join();
System.out.println("总共耗时:" + (System.currentTimeMillis() - start) / 1000 + " 分钟");
}
}
Promise
在多线程中,有一种被称为Promise
的模式,如果用这种模式来泡茶的话,就是这样的。
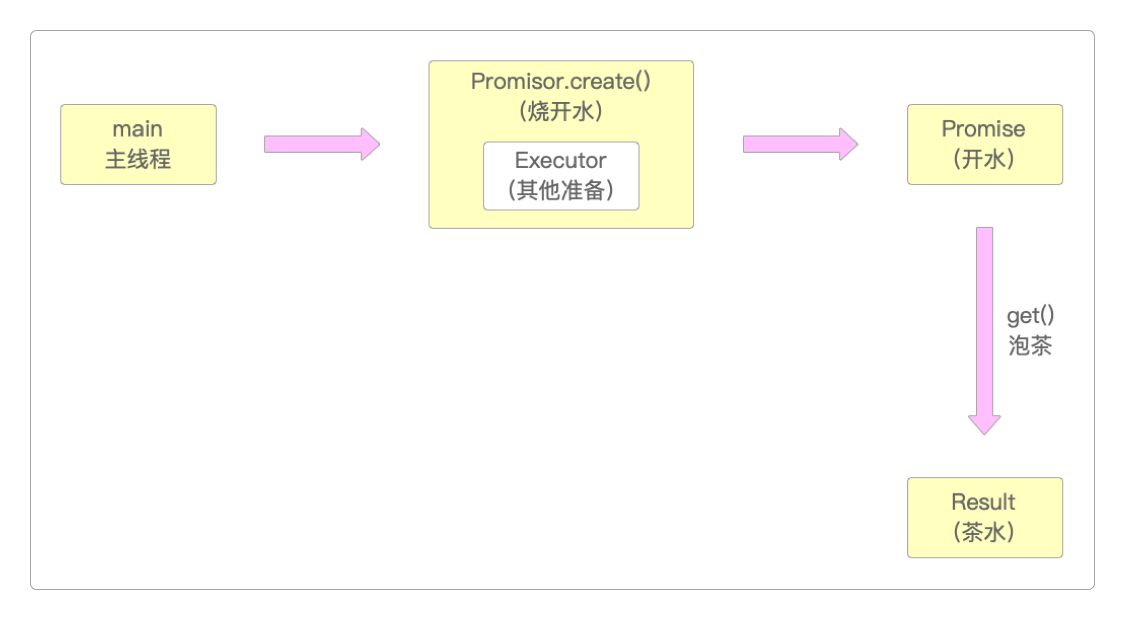
主线程调用
Promisor
类的create()
方法,这等于是在烧开水。在烧开水的同时,可以完成其他的任务
Executor
。水烧开了就可以得到
开水
,也就是Promise
。对
Promise
执行get()
方法(等于是泡茶
动作),就可以得到Result(茶水)
。
下面用代码来实现Promise
模式。
/**
* Promise模式泡茶
*
*/
public class Promisor {
/**
* 定义任务
*
*/
public static Future<Object> create(long start) {
// 烧开水
FutureTask<Object> futureTask = new FutureTask<>(() -> {
BoilWater bw = new BoilWater();
bw.setStatus(true);
TimeUnit.SECONDS.sleep(5);
System.out.println("开水烧好了,用时:" + (System.currentTimeMillis() - start) / 1000 + " 分钟");
return bw;
});
// 烧开水的同时执行其他工作,也就是Executor
new Thread(futureTask).start();
// 返回Promise
return futureTask;
}
public static void main(String[] args) throws InterruptedException, ExecutionException {
long start = System.currentTimeMillis();
// 获取Promise
Future<Object> promise = create(start);
// 执行准备工作
System.out.println("开始其他准备工作,需要5分钟,当前用时:" + (System.currentTimeMillis() - start) / 1000 + " 分钟");
OtherPreparation tc = new OtherPreparation();
tc.setStatus(true);
TimeUnit.SECONDS.sleep(2);
System.out.println("准备工作结束,等水烧开,用时:" + (System.currentTimeMillis() - start) / 1000 + " 分钟");
// 通过promise查看任务是否执行完毕
if (!promise.isDone()) {
System.out.println("还在等水烧开......");
}
// 通过promise来获取Result
BoilWater bw = (BoilWater) promise.get();
System.out.println("可以泡茶了,总共用时:" + (System.currentTimeMillis() - start) / 1000 + " 分钟");
}
}
感谢支持
更多内容,请移步《超级个体》。