登录功能
原创大约 3 分钟
创建模型类
创建src/main/ets/model/
目录,并创建ProfileInfo.ets
和UserInfo.ets
两个文件,内容如下。
/**
* 头像信息
*
*/
export class ProfileInfo {
// 头像编码
id: number = 0;
// 头像文件路径
path: string = "";
}
/**
* 用户信息
*
*/
export class UserInfo {
// 用户ID
userId: number = 0;
// 输入的账号
username: string = "";
// 输入的密码
password: string = "";
// 头像路径
profilePath: string = "";
// 构造函数
constructor(username: string, password: string, profilePath: string) {
this.username = username;
this.password = password;
this.profilePath = profilePath;
}
}
创建常量类
创建src/main/ets/common/
目录,并创建Constant.ets
文件,拷贝头像图片到src/main/resources/rawfile/profile
目录,Constant.ets
常量类中定义这些资源。
import { ProfileInfo } from "../model/ProfileInfo";
/**
* 常量类
*
*/
export default class Constant {
/**
* 路由参数
*/
static readonly ROUTER_PROFILE_INFO_ARRAY: string = "profileInfoArray";
/**
* 图片资源
*/
static readonly PROFILE_INFO_ARRAY: Array<ProfileInfo> = [
{ "id": 1, "path": "profile/profile_01.jpg" },
{ "id": 2, "path": "profile/profile_02.jpg" },
{ "id": 3, "path": "profile/profile_03.jpg" },
{ "id": 4, "path": "profile/profile_04.jpg" },
{ "id": 5, "path": "profile/profile_05.jpg" },
{ "id": 6, "path": "profile/profile_06.jpg" },
{ "id": 7, "path": "profile/profile_07.jpg" },
{ "id": 8, "path": "profile/profile_08.jpg" },
{ "id": 9, "path": "profile/profile_09.jpg" },
{ "id": 10, "path": "profile/profile_10.jpg" }
]
/**
* 头像路由参数
*/
static readonly ROUTER_SELECTED_PROFILE_INFO: string = "selectedProfileInfo";
/**
* 已经登录的用户路由参数
*/
static readonly ROUTER_LOGGED_USER_INFO: string = 'loggedUserInfo';
}
登录页面
/**
* 用户登录页面
*
*/
import router from '@ohos.router';
import Constant from '../common/Constant';
import { ProfileInfo } from '../model/ProfileInfo';
import { UserInfo } from '../model/UserInfo';
import { promptAction } from '@kit.ArkUI';
@Entry
@Component
struct Login {
@State
selectedProfileInfo: ProfileInfo = new ProfileInfo();
@State
profile: string = 'profile/user.png';
@State
username: string = "";
@State
password: string = "";
// 引入页面生命周期
onPageShow(): void {
let params = router.getParams();
if (null != params) {
this.selectedProfileInfo = (params as Record<string, ProfileInfo>)["selectedProfileInfo"];
this.profile = this.selectedProfileInfo.path;
}
}
@State
message: string = "登录页面";
build() {
// 先创建一个线性布局
Row() {
Column() {
// 显示头像
Image($rawfile(this.profile))
.width(100)
.height(100)
.borderRadius(5)
// 点击事件
.onClick(() => {
// 将头像JSON数据转为对象模型
router.pushUrl({
url: 'pages/ProfileAlbum',
params: { "profileInfoArray": Constant.PROFILE_INFO_ARRAY }
})
})
Row() {
Text("用户名:")
.width(80)
.margin({ top: 10 })
TextInput({placeholder: "请输入用户名"})
.width(220)
.height(50)
.borderRadius(5)
.backgroundColor('#e9e9e9')
.type(InputType.Normal)
.margin({ top: 10 })
.fontSize(25)
.onChange((value: string) => {
this.username = value;
})
}.margin({ top: 20 })
Row() {
Text("密 码:")
.width(80)
.margin({ top: 20 })
TextInput({placeholder: "请输入密码"})
.width(220)
.height(50)
.borderRadius(5)
.backgroundColor('#e9e9e9')
.type(InputType.Password)
.margin({ top: 20 })
.onChange((value: string) => {
this.password = value;
})
}
Button("登录", {type: ButtonType.Normal})
.width(280)
.margin({ top: 60})
.borderRadius(20)
.backgroundColor("#449EB7")
.fontSize(20)
.onClick(() => {
// 校验登录数据
if (this.username != "" && this.password !="" && this.selectedProfileInfo.path != null) {
// 校验通过
// 跳转到Index页面,同时传递用户登录信息
let userInfo: UserInfo = new UserInfo(this.username, this.password, this.selectedProfileInfo.path);
router.replaceUrl({
url: 'pages/Index',
params: { "loggedUserInfo": userInfo }
})
} else {
// 校验不通过
promptAction.showToast({
message: '账号、密码、头像不能为空!',
duration: 2000
});
}
})
}.width("100%")
}.height("100%")
}
}
头像相册
在src/main/ets/pages/
目录中创建ProfileAlbum.ets
文件,内容如下。
/**
* 头像相册
*
*/
import { ProfileInfo } from '../model/ProfileInfo'
import { router } from '@kit.ArkUI'
import Constant from '../common/Constant';
@Entry
@Component
struct ProfileAlbum {
// 从路由对象中获取到头像数组
@State profileInfoArray: Array<ProfileInfo> = (router.getParams() as Record<string, Array<ProfileInfo>>) [`${Constant.ROUTER_PROFILE_INFO_ARRAY}`];
build() {
// 导航组件,用于返回
Navigation() {
// 网格组件
Grid() {
// 循环添加网格项目GridItem
ForEach(this.profileInfoArray, (profileInfo: ProfileInfo) => {
GridItem() {
Image($rawfile(profileInfo.path)).width('100%').height('100%')
// 实现点击事件
.onClick(() => {
router.back({
url: 'pages/Login',
params: {"selectedProfileInfo": profileInfo}
})
})
}
.width('100%')
// 头像宽高比1:1
.aspectRatio(1)
})
}
.columnsTemplate('1fr 1fr 1fr 1fr')
// 行间距和列间距
.rowsGap(5).columnsGap(5)
}
.title("头像选择")
// 显示返回按钮
.hideBackButton(false)
.titleMode(NavigationTitleMode.Mini)
}
}
更新路由
在src/main/resources/base/profile/main_pages.json
中更新路由。
{
"src": [
"pages/Index",
"pages/Login",
"pages/ProfileAlbum"
]
}
最终的预览效果如下图所示。
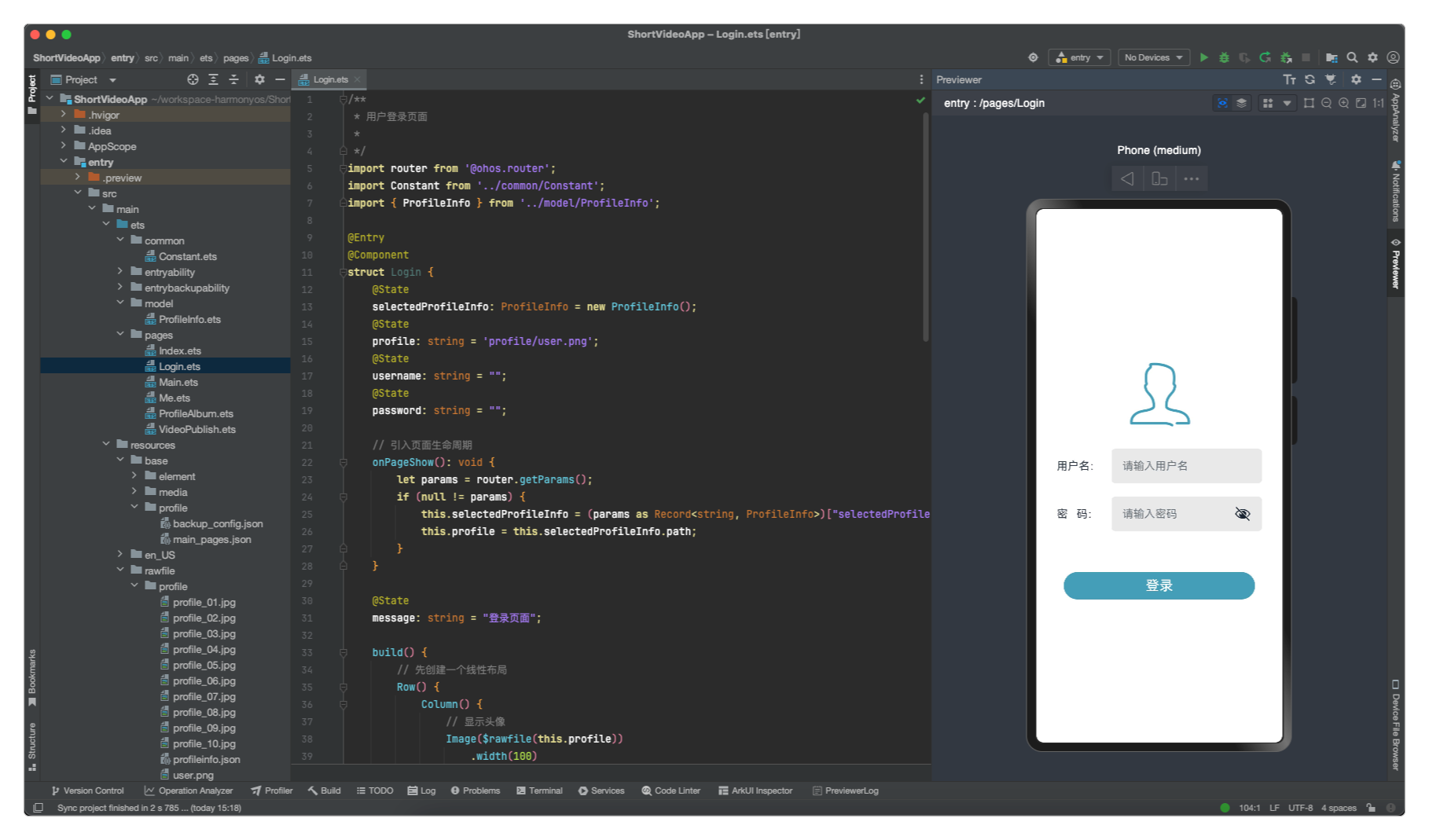
感谢支持
更多内容,请移步《超级个体》。