视频发布
原创大约 4 分钟
定义常量数据
在src/main/ets/common/Constant.ets
文件中增加相关的常量数据。
这里的数据都是用来模拟从本地选择视频文件时的效果。
/**
* 常量类
*
*/
export default class Constant {
......
/**
* 待上传视频数据
*/
static readonly VIDEO_INFO_WAIT_UPLOAD_ARRAY: Array<VideoInfo> = [
{
"videoId": 6,
"coverPath": "video/video_cover_06.jpg",
"videoPath": "video/video_06.mp4",
"author": "",
"profilePath": "",
"content": "",
"thumbsUpCount": 0,
"commentCount": 0,
"favoriteCount": 0,
"shareCount": 0,
"isFollow": false,
"isThumbsUp": false,
"isFavorite": false
},
{
"videoId": 7,
"coverPath": "video/video_cover_07.jpg",
"videoPath": "video/video_07.mp4",
"author": "",
"profilePath": "",
"content": "",
"thumbsUpCount": 0,
"commentCount": 0,
"favoriteCount": 0,
"shareCount": 0,
"isFollow": false,
"isThumbsUp": false,
"isFavorite": false
},
{
"videoId": 8,
"coverPath": "video/video_cover_08.jpg",
"videoPath": "video/video_08.mp4",
"author": "",
"profilePath": "",
"content": "",
"thumbsUpCount": 0,
"commentCount": 0,
"favoriteCount": 0,
"shareCount": 0,
"isFollow": false,
"isThumbsUp": false,
"isFavorite": false
},
{
"videoId": 9,
"coverPath": "video/video_cover_09.jpg",
"videoPath": "video/video_09.mp4",
"author": "",
"profilePath": "",
"content": "",
"thumbsUpCount": 0,
"commentCount": 0,
"favoriteCount": 0,
"shareCount": 0,
"isFollow": false,
"isThumbsUp": false,
"isFavorite": false
},
{
"videoId": 10,
"coverPath": "video/video_cover_10.jpg",
"videoPath": "video/video_10.mp4",
"author": "",
"profilePath": "",
"content": "",
"thumbsUpCount": 0,
"commentCount": 0,
"favoriteCount": 0,
"shareCount": 0,
"isFollow": false,
"isThumbsUp": false,
"isFavorite": false
}
];
/**
* 已选择的视频数据
*/
static readonly SELECTED_VIDEO_INFO: string = 'selectedVideoInfo';
/**
* 待发布的视频数据
*/
static readonly WAIT_PUBLISHED_VIDEO_INFO: string = 'waitPublishVideoInfo';
}
创建发布界面
在src/main/ets/pages/
目录中创建VideoPublish.ets
文件,内容如下。
import router from "@ohos.router";
import { VideoInfo } from "../model/VideoInfo";
import promptAction from "@ohos.promptAction";
/**
* 视频发布
*
*/
@Entry
@Component
export struct VideoPublish {
@State
selectedVideoInfo: VideoInfo = new VideoInfo();
onPageShow(): void {
// 获取选中的视频封面
let params = router.getParams();
if (params != null) {
this.selectedVideoInfo = (params as Record<string, VideoInfo>)["selectedVideoInfo"];
}
}
build() {
// 导航组件,用于返回
Navigation() {
Row() {
Column() {
// 视频封面
Image($rawfile(this.selectedVideoInfo.coverPath != "" ? this.selectedVideoInfo.coverPath : "video/video_upload.png"))
.width(145)
.height(175)
// 点击跳转到视频相册
.onClick(() => {
router.pushUrl({
url: 'pages/VideoAlbum',
})
})
// 视频内容
TextArea({ placeholder: '请输入视频内容介绍' })
.width(320)
.height(100)
.borderRadius(5)
.backgroundColor('#e3e3e3')
.margin({ top: 15 })
.fontSize(20)
// 保存输入的内容
.onChange((value: string) => {
this.selectedVideoInfo.content = value;
})
// 发布按钮
Button('发布', { type: ButtonType.Normal })
.width(320)
.height(50)
.borderRadius(8)
.fontSize(24)
.margin({ top: 20 })
// 校验输入的内容
.onClick(() => {
if (this.selectedVideoInfo.coverPath != "" && this.selectedVideoInfo.content != "") {
// 获取AppStorage中保存的用户信息
let username: string | undefined = AppStorage.get("UserInfo.username");
let profilePath: string | undefined = AppStorage.get("UserInfo.profilePath");
// 将用户信息设置到选中的视频信息中去
this.selectedVideoInfo.author = username as string;
this.selectedVideoInfo.profilePath = profilePath as string;
router.replaceUrl({
url: 'pages/Index',
// 通过路由参数传递待发布的视频
params: {
"waitPublishVideoInfo": this.selectedVideoInfo
}
})
} else {
promptAction.showToast({
message: '视频封面和内容不能为空',
duration: 2000
})
}
})
}.width("100%")
}.height("100%")
}
.title('发布视频')
.hideBackButton(false)
.titleMode(NavigationTitleMode.Mini)
}
}
创建视频相册
在src/main/ets/pages/
目录中创建VideoAlbum.ets
文件,内容如下。
import { VideoInfo } from '../model/VideoInfo';
import Constant from '../common/Constant';
import router from '@ohos.router';
/**
* 视频相册
*
*/
@Entry
@Component
struct VideoAlbum {
build() {
// 导航组件,用于返回
Navigation() {
// 网格组件
Grid() {
// 循环添加网格项目GridItem
ForEach(Constant.VIDEO_INFO_WAIT_UPLOAD_ARRAY, (videoInfo: VideoInfo) => {
GridItem() {
Image($rawfile(videoInfo.coverPath))
.width('100%').height('100%')
// 选择视频封面
.onClick(() => {
router.back({
url: 'pages/VideoPublish',
params: {
"selectedVideoInfo": videoInfo
}
})
})
}
.width('100%')
// 宽高比
.aspectRatio(0.755)
})
}
.columnsTemplate('1fr 1fr 1fr 1fr')
// 行间距和列间距
.rowsGap(5).columnsGap(5)
}
.title('视频相册')
.hideBackButton(false)
.titleMode(NavigationTitleMode.Mini)
}
}
修改首页
修改后的src/main/ets/pages/Index.ets
首页代码如下。
import Constant from '../common/Constant';
import { VideoInfoDataSource } from '../datasource/VideoInfoDataSource';
import { Main } from './Main'
import { Me } from './Me'
import router from '@ohos.router';
import { UserInfo } from '../model/UserInfo';
import { VideoInfo } from '../model/VideoInfo';
/**
* 主界面
*
*/
@Entry
@Component
struct Index {
// 是否显示视频界面
@State
isShow: boolean = false;
// 视频信息数据源
@State
videoInfoDataSource: VideoInfoDataSource = new VideoInfoDataSource(Constant.VIDEO_INFO_ARRAY);
// 当前Tabs页面的索引
@State
currentIndex: number = 0;
// TabsController组件
private tabsController: TabsController = new TabsController();
// 在其他生命周期处理isShow变量
aboutToAppear(): void {
this.isShow = true;
}
aboutToDisappear(): void {
this.isShow = false;
}
onPageShow(): void {
this.isShow = true;
// 从路由参数中获取用户信息
let params = router.getParams();
if (params != null) {
let userInfo: UserInfo = (params as Record<string, UserInfo>)[`${Constant.ROUTER_LOGGED_USER_INFO}`];
if (userInfo != null) {
// 存储在AppStorage中
AppStorage.setOrCreate('UserInfo.username', userInfo.username);
AppStorage.setOrCreate('UserInfo.profilePath', userInfo.profilePath);
}
// 从路由参数中获取视频信息
let selectedVideoInfo: VideoInfo = (params as Record<string, VideoInfo>)[`${Constant.WAIT_PUBLISHED_VIDEO_INFO}`];
if (selectedVideoInfo != null) {
// 存储至视频数据源
this.videoInfoDataSource.push(selectedVideoInfo)
}
}
}
onPageHide(): void {
this.isShow = false;
}
build() {
// 设置导航栏和Tabs的控制器
Tabs({ barPosition: BarPosition.End, controller: this.tabsController }) {
// 各个导航的页签
TabContent() {
Main({
isShow: this.isShow,
videoInfoDataSource: this.videoInfoDataSource
})
}.tabBar(this.TabBuilder("首页", 0))
TabContent() {
// VideoPublish()
}.tabBar(this.TabBuilder("发布", 1))
TabContent() {
Me()
}.tabBar(this.TabBuilder("我", 2))
}
}
/**
* 自定义TabBuilder导航栏组件
*
*/
@Builder
TabBuilder(title: string, index: number) {
Column() {
// 设置选中状态
Text(title)
.fontColor(this.currentIndex === index ? '#FFFFFF' : '#999999')
}
.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
.backgroundColor(Color.Black)
// 设置点击事件
.onClick(() => {
this.currentIndex = index;
// 页面的索引从0开始
if (index === 0) {
this.tabsController.changeIndex(index);
this.isShow = true;
} else {
this.isShow = false;
}
// 如果是点击视频发布
if (index === 1) {
router.pushUrl({
url: 'pages/VideoPublish'
})
}
// 如果是点击“我”
if (index === 2) {
this.tabsController.changeIndex(index);
}
})
}
}
修改完成后,通过登录
-> 发布视频
-> 输入视频信息
-> 确定
,然后在首页上一直向下滑动,最后一条视频显示的就是刚才发布的内容。
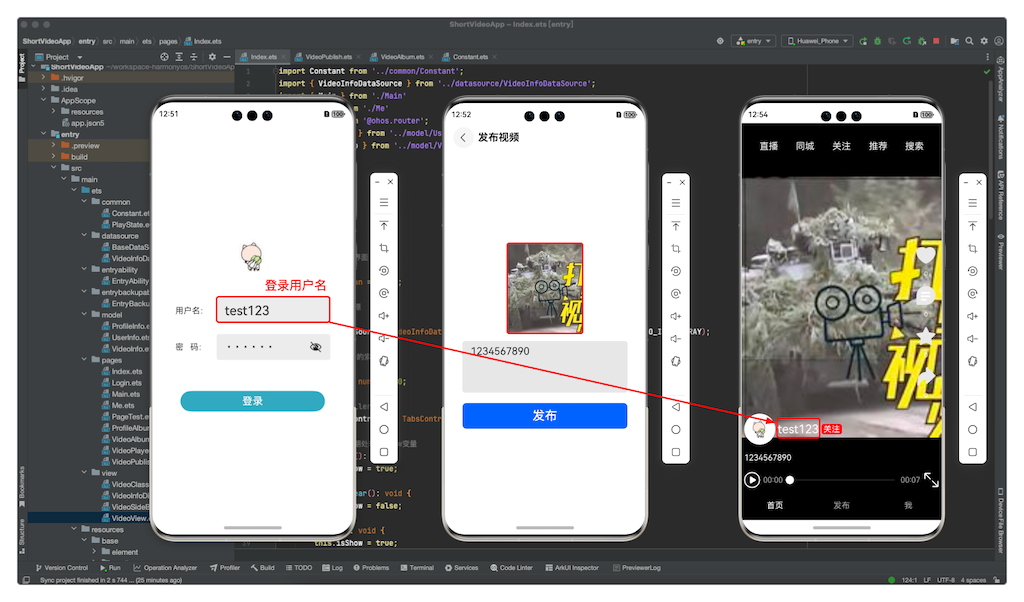
感谢支持
更多内容,请移步《超级个体》。