社交元素
原创大约 4 分钟
本质上,点赞
、关注
、收藏
这三连
的逻辑都是一样的,所以就只以点赞
为例来说明。
点赞数据源
在src/main/ets/datasource/
目录中创建LikeDataSource.ets
文件,内容如下。
import { VideoInfo } from '../model/VideoInfo';
import { BaseDataSource } from './BaseDataSource';
/**
* 点赞信息数据源
*
*/
export class LikeDataSource extends BaseDataSource<VideoInfo> {
constructor(videoArray: Array<VideoInfo>) {
super(videoArray)
}
/**
* 是否已经点赞过
*/
existLike(id: number): boolean {
let dataSource: Array<VideoInfo> = this.getDataSource();
// 遍历数组
for (let i: number = 0; i < dataSource.length; i++) {
if (dataSource[i].videoId === id) {
return true;
}
}
return false;
}
/**
* 根据ID删除数据
*/
removeById(id: number): void {
let dataSource: Array<VideoInfo> = this.getDataSource();
// 遍历数据并删除
for (let i: number = 0; i < dataSource.length; i++) {
if (dataSource[i].videoId === id) {
this.remove(i);
break;
}
}
}
}
点赞列表
在src/main/ets/view/
目录中创建MeLikeList.ets
文件,内容如下。
import { LikeDataSource } from "../datasource/LikeDataSource";
import { VideoInfo } from "../model/VideoInfo";
import { JSON } from "@kit.ArkTS";
import { VideoInfoDataSource } from "../datasource/VideoInfoDataSource";
/**
* “我”页面点赞列表
*
*/
@Component
export struct MeLikeList {
// 视频数据源
@Consume
videoInfoDataSource: VideoInfoDataSource
// 点赞数据源
@Consume
likeDataSource: LikeDataSource;
build() {
Column() {
List({ space: 5 }) {
// 生成列表项
LazyForEach(
this.likeDataSource,
// 列表项itemGenerator
(videoInfo: VideoInfo) => {
ListItem() {
Row() {
// 视频封面
Image($rawfile(videoInfo.coverPath))
.width(136)
.height(180)
// 视频文本
Text(videoInfo.content)
.width(160)
.fontSize(16)
.fontColor('#FFFFFF')
// 操作按钮
Column() {
Button('删除', { type: ButtonType.Normal })
.width(80)
.height(24)
.borderRadius(4)
.fontSize(14)
.backgroundColor(Color.Red)
.onClick(() => {
// 从数据源中删除
this.likeDataSource.removeById(videoInfo.videoId)
// 取消点赞
videoInfo.isThumbsUp = false;
// 点赞量减1
videoInfo.thumbsUpCount = videoInfo.thumbsUpCount - 1;
// 更新视频数据源
this.videoInfoDataSource.updateById(videoInfo.videoId, videoInfo)
})
}
.width(80)
}
.width('100%')
.height(180)
}
.width('100%')
},
// 列表关键字keyGenerator
(videoInfo: VideoInfo) => JSON.stringify(videoInfo)
)
}
.width('100%')
.listDirection(Axis.Vertical)
.edgeEffect(EdgeEffect.Spring)
}
.width('100%')
.height('100%')
}
}
加入到分类标签
将点赞列表页面加入到分类标签MeClassification.ets
中。
import { UserVideoDataSource } from "../datasource/UserVideoDataSource";
import { MeLikeList } from "./MeLikeList";
import { MeVideoList } from "./MeVideoList";
/**
* “我”作品分类
*
*/
@Component
export struct MeClassification {
// 当前Tabs页签
@State
currentIndex: number = 0;
// 用户上传的视频数据源
@Link
userVideoDataSource: UserVideoDataSource;
build() {
Column() {
// 设置Tabs组件
Tabs({ barPosition: BarPosition.Start }) {
TabContent() {
// 作品列表
MeVideoList({
userVideoDataSource: this.userVideoDataSource
})
}.tabBar(this.TabBuilder('作品', 0))
TabContent() {
// 点赞列表
MeLikeList()
}.tabBar(this.TabBuilder('喜欢', 1))
TabContent() {
// 收藏列表的实现与点赞类似
}.tabBar(this.TabBuilder('收藏', 2))
TabContent() {
// 关注列表的实现与点赞类似
}.tabBar(this.TabBuilder('关注', 3))
}
.onChange((index: number) => {
this.currentIndex = index;
})
}
.width('100%')
.height('100%')
}
/**
* 自定义导航栏
*/
@Builder
TabBuilder(title: string, index: number) {
Column() {
// 选中和不选中的文本样式
Text(title)
.fontColor(this.currentIndex === index ? '#FFFFFF' : '#F8FAEE')
.fontSize(this.currentIndex === index ? 20 : 18)
// 选中时下划线
Divider()
.strokeWidth(1)
.color('#FFFFFF')
.visibility(this.currentIndex === index ? Visibility.Visible : Visibility.Hidden)
}
.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
}
}
侧边栏事件
在侧边栏VideoSideBar.ets
组件中实现点赞和取消点赞的鼠标单击事件。
import { FavoriteDataSource } from "../datasource/FavoriteDataSource";
import { LikeDataSource } from "../datasource/LikeDataSource";
import { VideoInfoDataSource } from "../datasource/VideoInfoDataSource";
import { VideoInfo } from "../model/VideoInfo"
/**
* 视频侧边栏组件
*
*/
@Component
export struct VideoSideBar {
// 视频数据源
@Consume
videoInfoDataSource: VideoInfoDataSource
// 点赞数据源
@Consume
likeDataSource: LikeDataSource;
// 收藏数据源
@Consume
favoriteDataSource: FavoriteDataSource;
@State
videoInfo: VideoInfo = new VideoInfo();
aboutToAppear(): void {
// 判读是否点赞
this.videoInfo.isThumbsUp = this.likeDataSource.existLike(this.videoInfo.videoId)
// 判读是否收藏
this.videoInfo.isFavorite = this.favoriteDataSource.existFavorite(this.videoInfo.videoId)
}
build() {
Column() {
/**
* 点赞组件
*/
// 点赞按钮
Image(this.videoInfo.isThumbsUp ? $r('app.media.like_red') : $r('app.media.like'))
.width(40)
.height(40)
// 点赞及取消点赞
.onClick(() => {
if (this.videoInfo.isThumbsUp) {
// 已点赞则取消点赞
this.videoInfo.isThumbsUp = false;
// 点赞量减1
this.videoInfo.thumbsUpCount = this.videoInfo.thumbsUpCount - 1;
// 从数据源中删除
this.likeDataSource.removeById(this.videoInfo.videoId)
} else {
// 未点赞过则点赞
this.videoInfo.isThumbsUp = true;
// 点赞量加1
this.videoInfo.thumbsUpCount = this.videoInfo.thumbsUpCount + 1;
// 增加到数据源
this.likeDataSource.push(this.videoInfo)
}
// 将点赞量更新到视频数据源
this.videoInfoDataSource.updateById(this.videoInfo.videoId, this.videoInfo)
})
// 点赞数量
Text(this.videoInfo.thumbsUpCount + '')
.margin({ top: 10 })
.fontSize(12)
.fontColor('#FFFFFF')
.width(40)
.textAlign(TextAlign.Center)
/**
* 评论组件
*/
// 评论按钮
Image($r('app.media.comment'))
.width(40)
.height(40)
.margin({ top: 15 })
// 评论数量
Text(this.videoInfo.commentCount + '')
.margin({ top: 10 })
.fontSize(12)
.fontColor('#FFFFFF')
.width(40)
.textAlign(TextAlign.Center)
/**
* 收藏组件
*/
// 收藏按钮
Image(this.videoInfo.isFavorite ? $r('app.media.star_red') : $r('app.media.star'))
.width(40)
.height(40)
.margin({ top: 15 })
// 收藏数量
Text(this.videoInfo.favoriteCount + '')
.margin({ top: 10 })
.fontSize(12)
.fontColor('#FFFFFF')
.width(40)
.textAlign(TextAlign.Center)
/**
* 分享组件
*/
// 分享按钮
Image($r('app.media.share'))
.width(40)
.height(40)
.margin({ top: 15 })
// 分享数量
Text(this.videoInfo.shareCount + '')
.margin({ top: 10 })
.fontSize(12)
.fontColor('#FFFFFF')
.width(40)
.textAlign(TextAlign.Center)
}
.width(40)
.height(260)
}
}
其他
最后在Index.ets
中增加对视频信息数据源
和点赞数据源
的调用。
@Entry
@Component
struct Index {
......
// 视频信息数据源
@Provide
videoInfoDataSource: VideoInfoDataSource = new VideoInfoDataSource(Constant.VIDEO_INFO_ARRAY);
// 点赞数据源
@Provide
likeDataSource: LikeDataSource = new LikeDataSource(Constant.LIKE_INFO_ARRAY);
......
}
记得在常量类Constant.ets
中增加相关常量。
export default class Constant {
......
/**
* 点赞的数据
*/
static LIKE_INFO_ARRAY: Array<VideoInfo> = [];
}
最终的预览效果如下图所示。
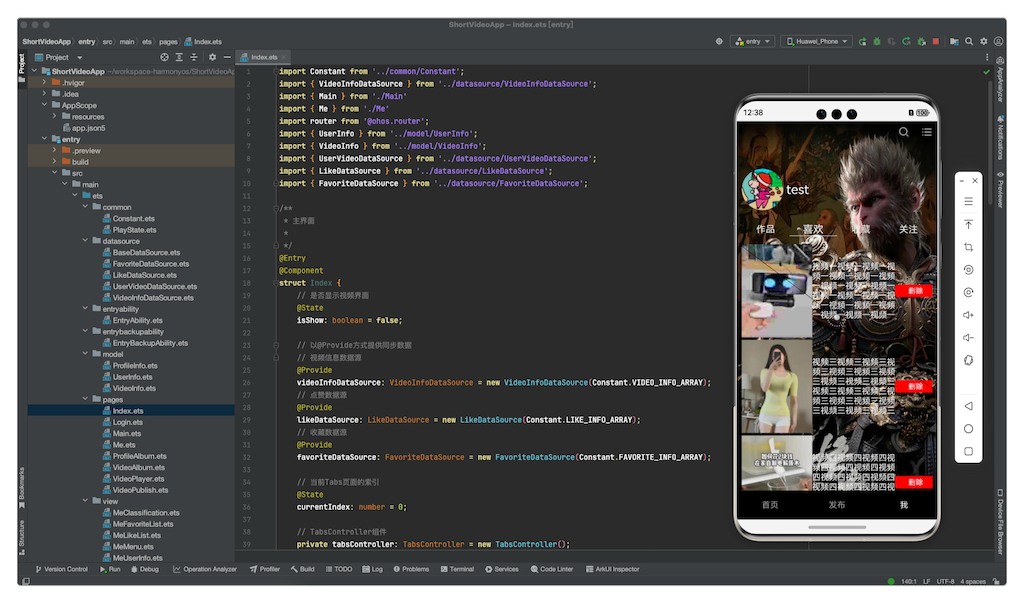
感谢支持
更多内容,请移步《超级个体》。