UI界面
PyQt
> pip install pyqt6
> pip install pyqt6-tools
注意:目前pyqt6
和pyqt6-tools
只能在Python3.9
版本中安装成功,用最新的版本中可能会出现依赖冲突。
然后,在PyCharm中添加外部工具。
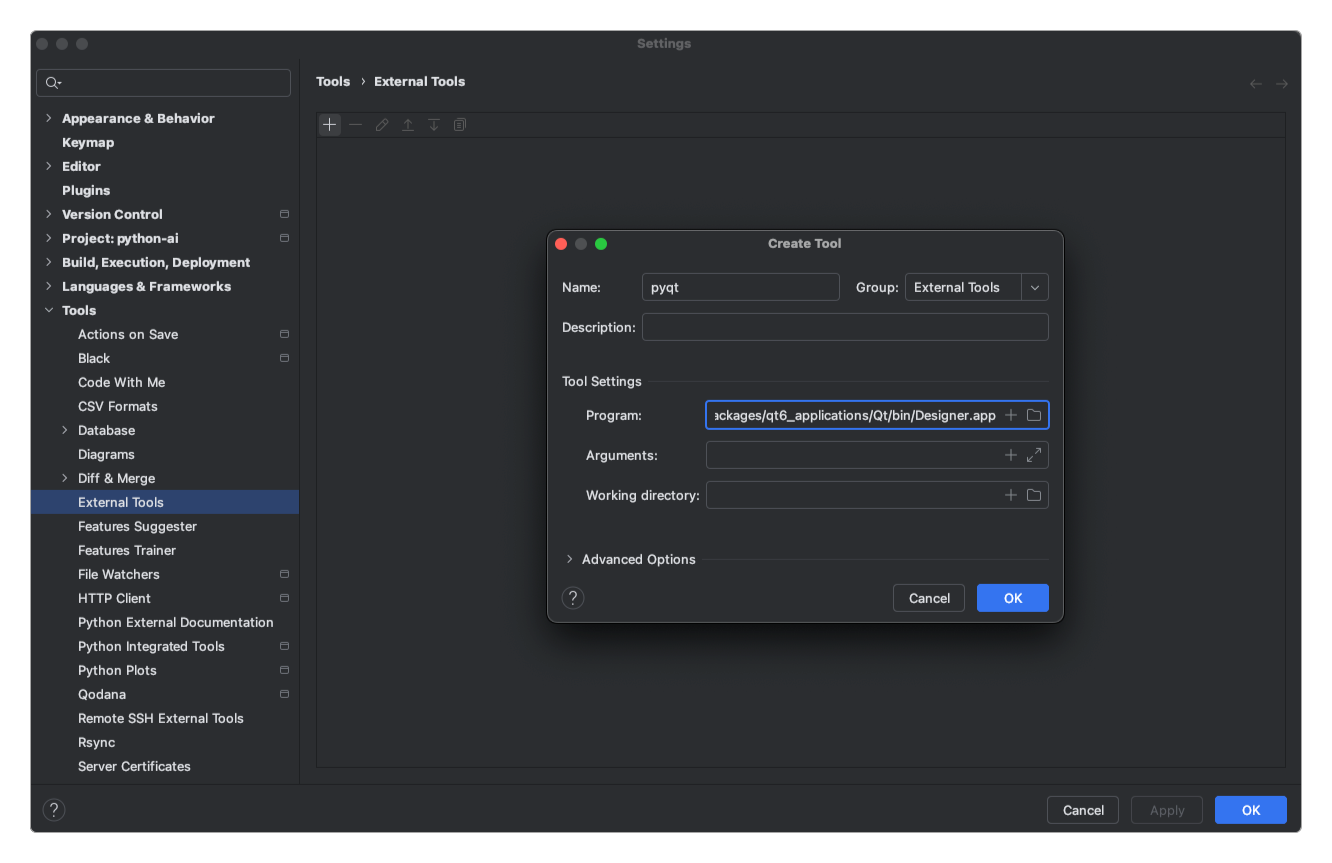
此处路径为${anaconda3}/envs/dev-3.9/lib/python3.9/site-packages/qt6_applications/Qt/bin/Designer.app
。
创建新的Python文件,然后单击鼠标右键,在弹出的菜单中选择External Tools
-> pyqt
。
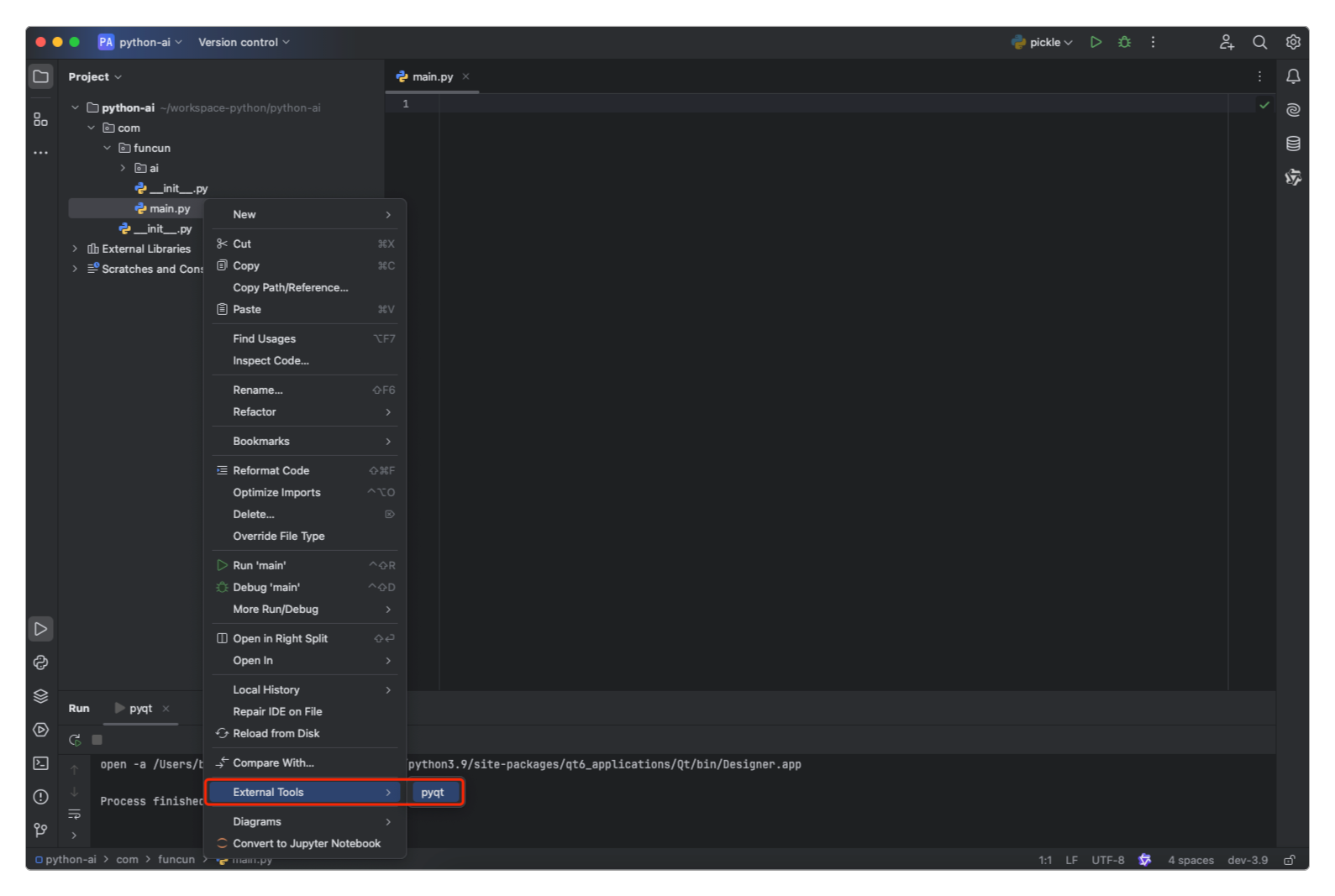
打开后,就会出现如下的PyQt界面。
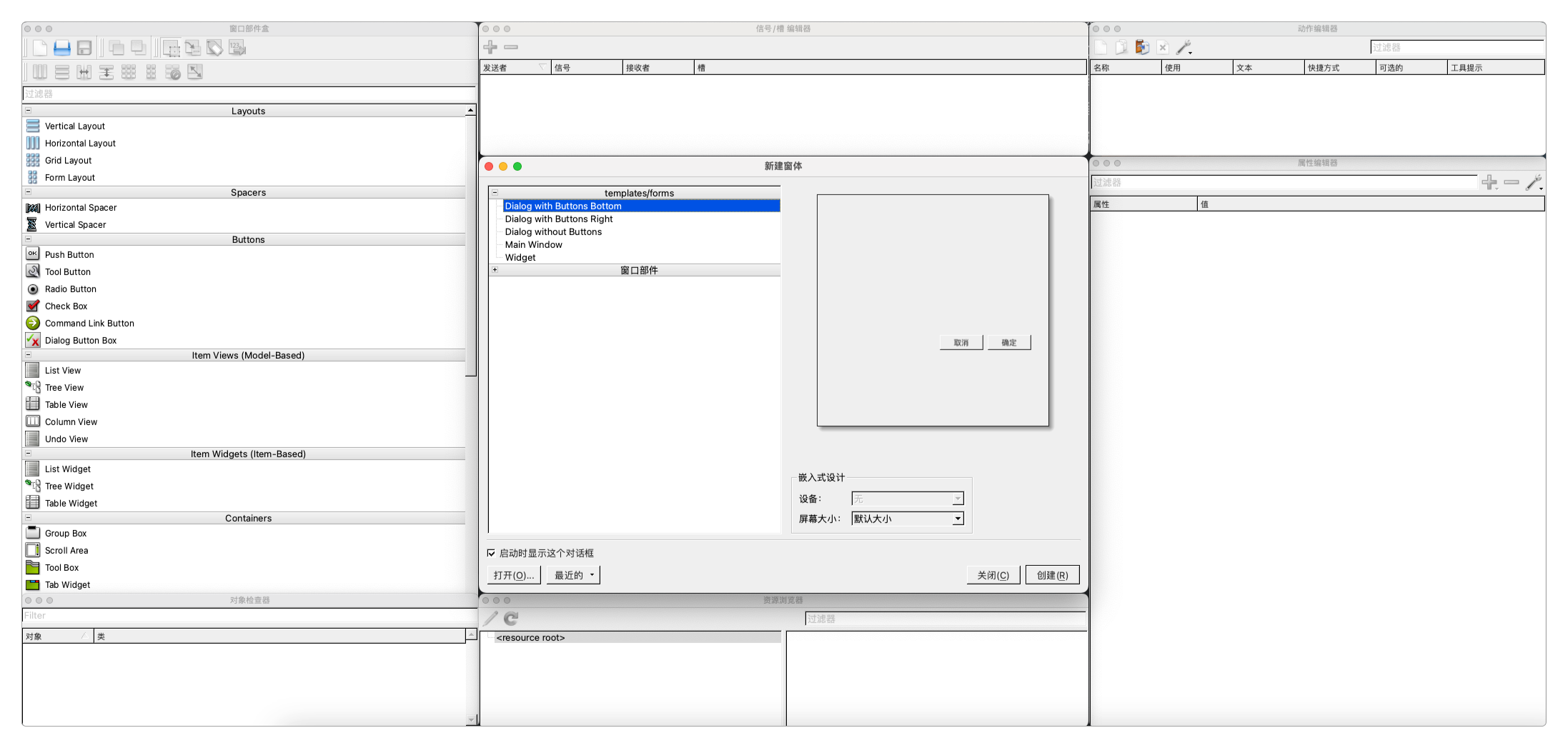
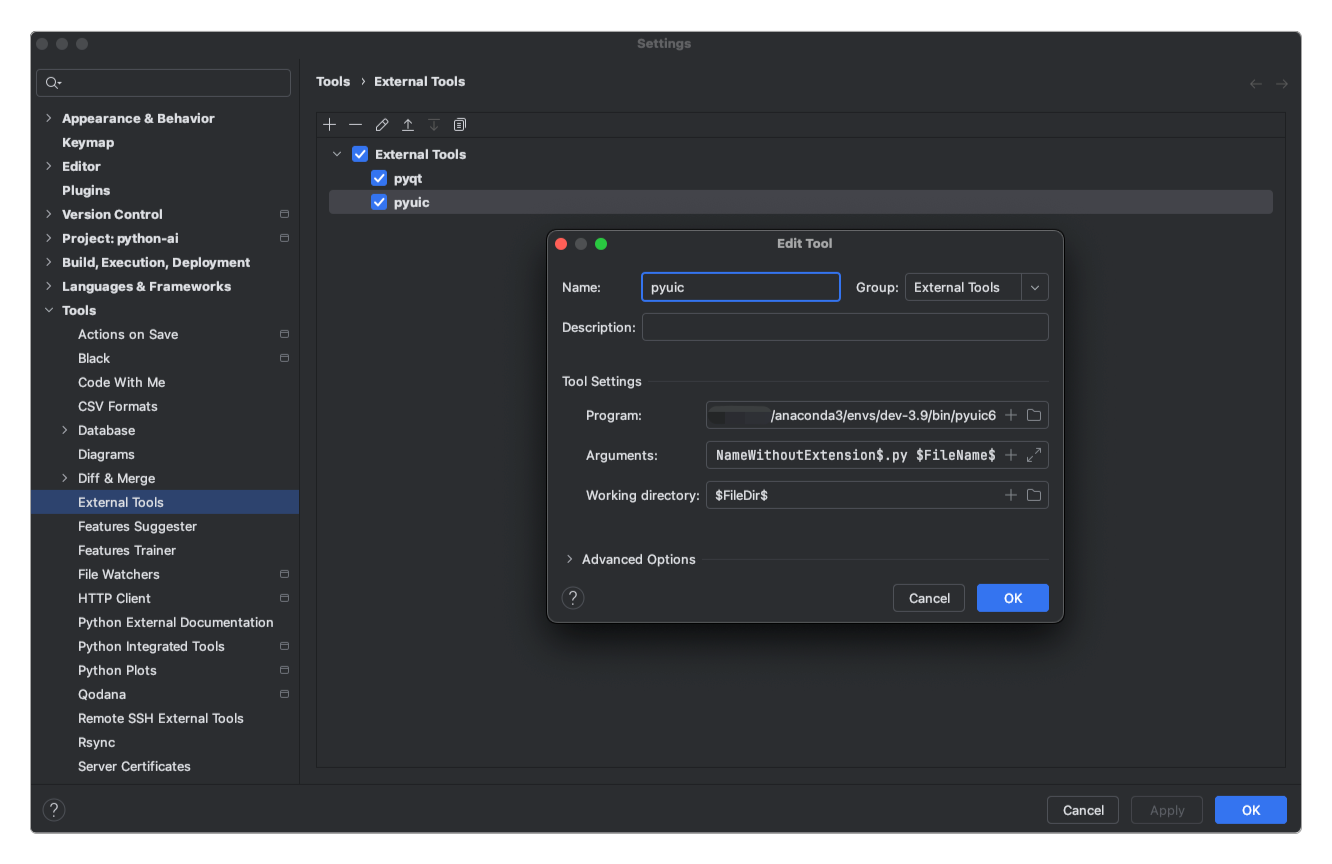
输入的内容分别如下。
Program
:${anaconda3}/envs/dev-3.9/bin/pyuic6
。Arguments
:-o $FileNameWithoutExtension$.py $FileName$
。Working dirctory
:$FileDir$
。
在PyQt界面新建一个MainWindow
,将它保存到和main.py
相同的目录中,并命名为qttest.ui
。
在qttest.ui
文件上单击鼠标右键,通过pyuic来自动生成对应的qttest.py
文件。
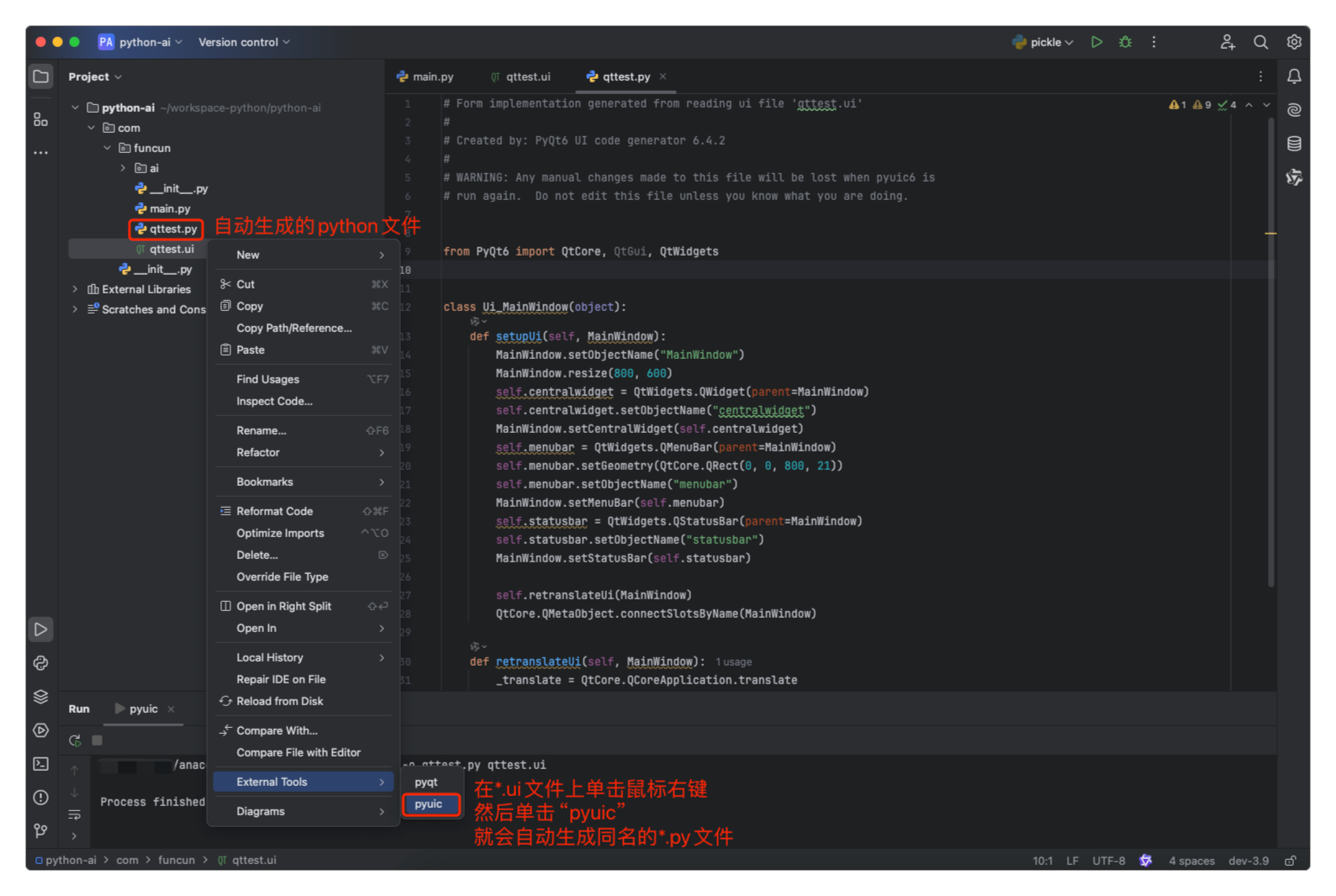
之后在main.py
中输入如下代码。
from qttest import Ui_MainWindow
import sys
from PyQt6.QtWidgets import QApplication, QMainWindow
class TestWindow(QMainWindow, Ui_MainWindow):
def __init__(self, parent=None):
super(TestWindow, self).__init__(parent)
self.setupUi(self)
if __name__ == '__main__':
app = QApplication(sys.argv)
ui = TestWindow()
ui.show()
sys.exit(app.exec())
运行后出现可视化界面。
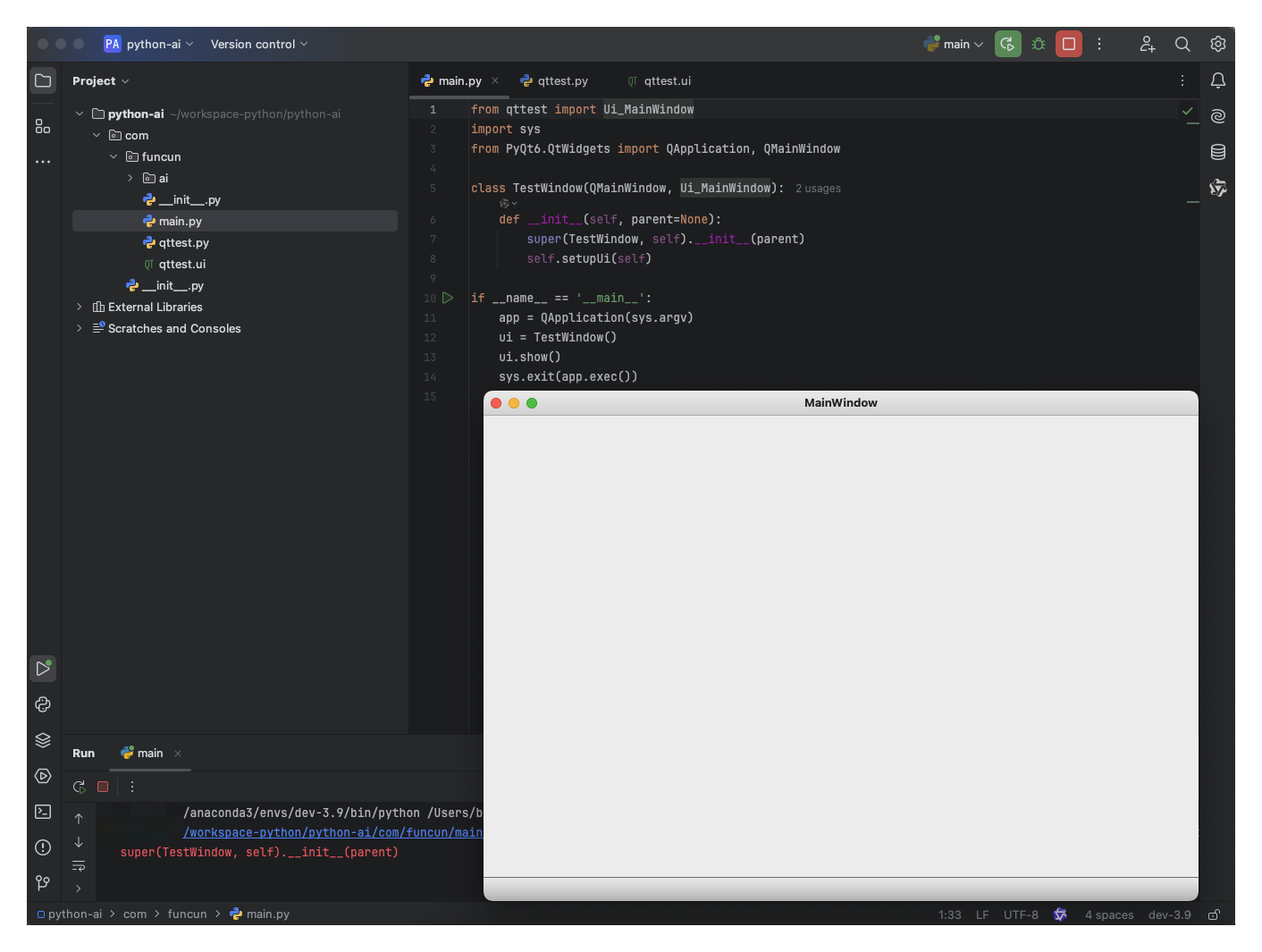
用PyQt重新打开qttest.ui
文件,然后拖入PushButton
和Label
两个组件到窗口中,分别命名为imageLabel
和openFileButton
。
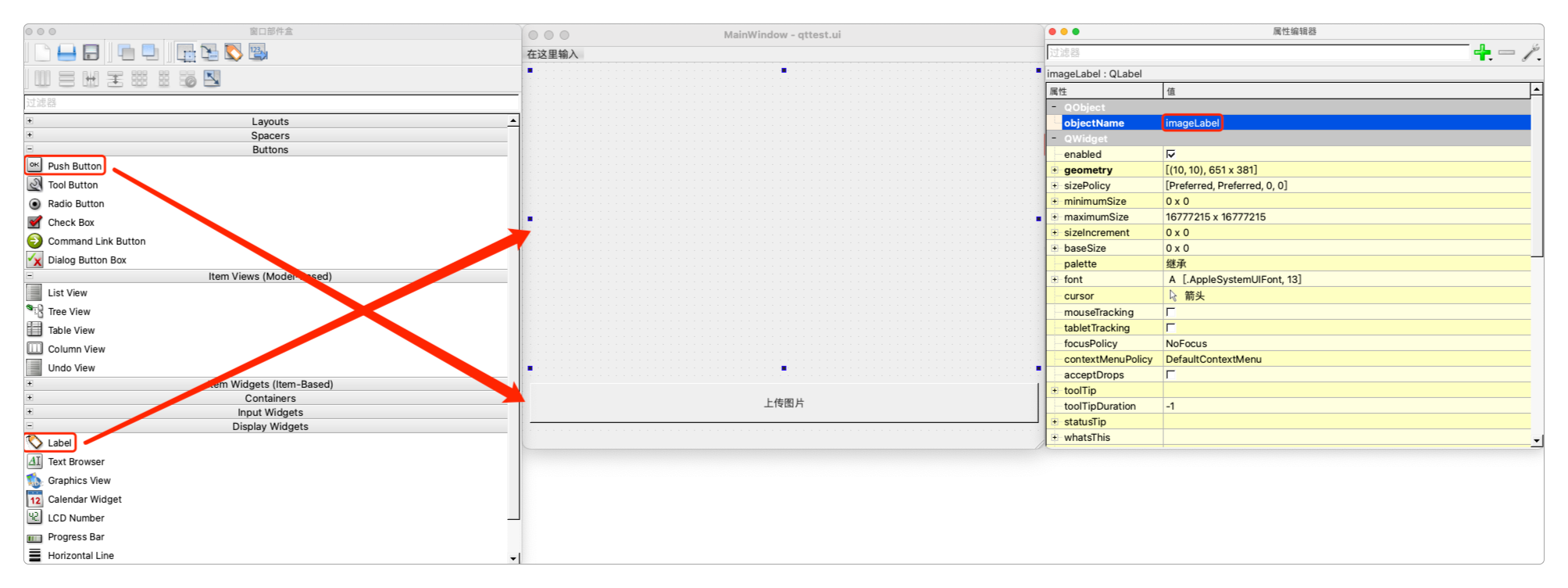
之后用pyuic重新生成对应的qttest.py
文件。
在main.py
添加实现功能的代码。
from qttest import Ui_MainWindow
import sys
from PyQt6.QtWidgets import QApplication, QMainWindow, QFileDialog
from PyQt6 import QtGui
class TestWindow(QMainWindow, Ui_MainWindow):
def __init__(self, parent=None):
super(TestWindow, self).__init__(parent)
self.setupUi(self)
self.openFileButton.clicked.connect(self.openFile)
def openFile(self):
self.filename, _ = QFileDialog.getOpenFileName(self, "打开文件", ".", "图片文件(*.jpg *.png)")
pixmap = QtGui.QPixmap(self.filename).scaled(self.imageLabel.width(), self.imageLabel.height())
self.imageLabel.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication(sys.argv)
ui = TestWindow()
ui.show()
sys.exit(app.exec())
运行后效果如下图。
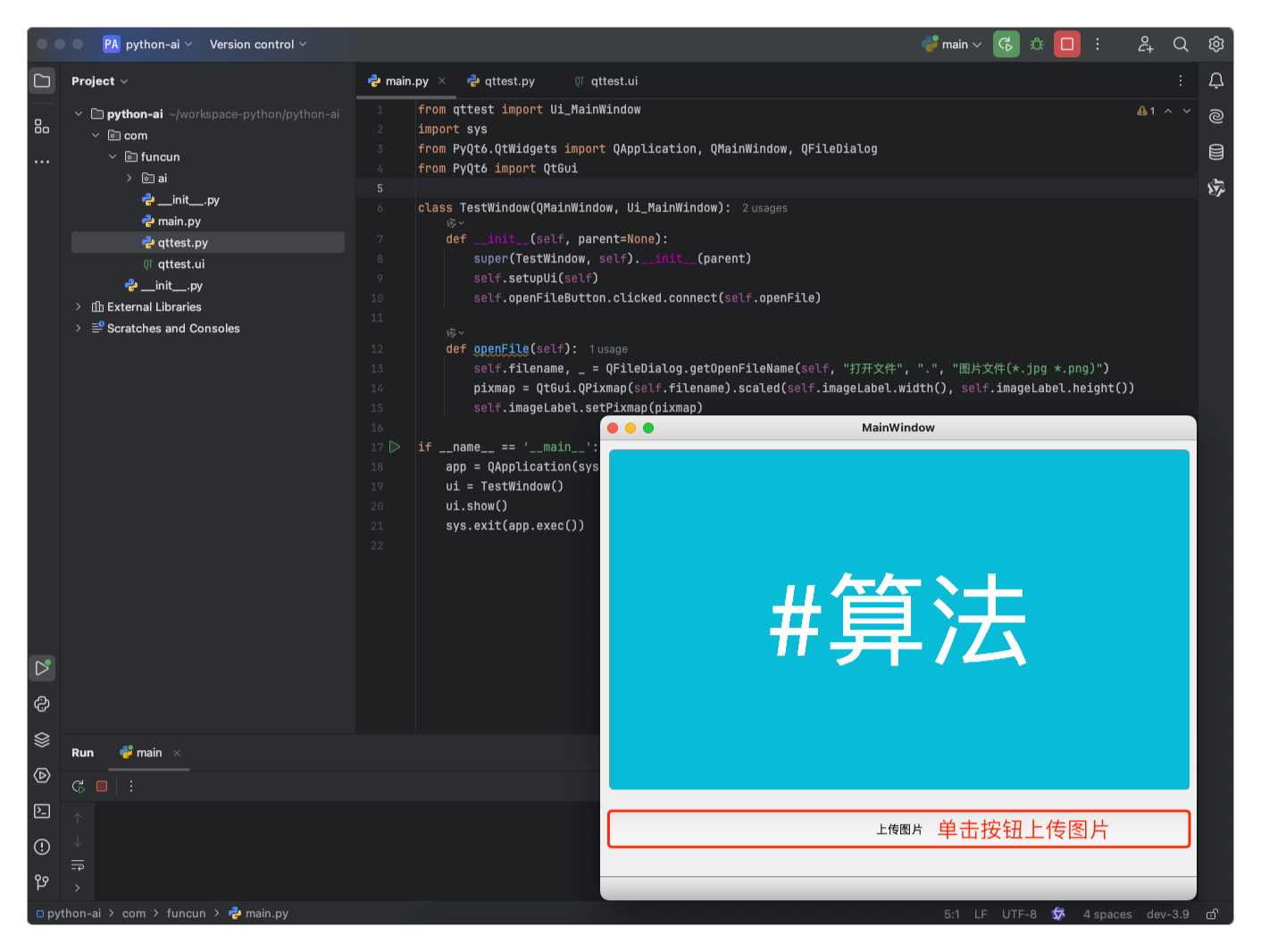
Gradio
大名鼎鼎的Stable Diffusion就是通过Gradio实现文生图应用的。
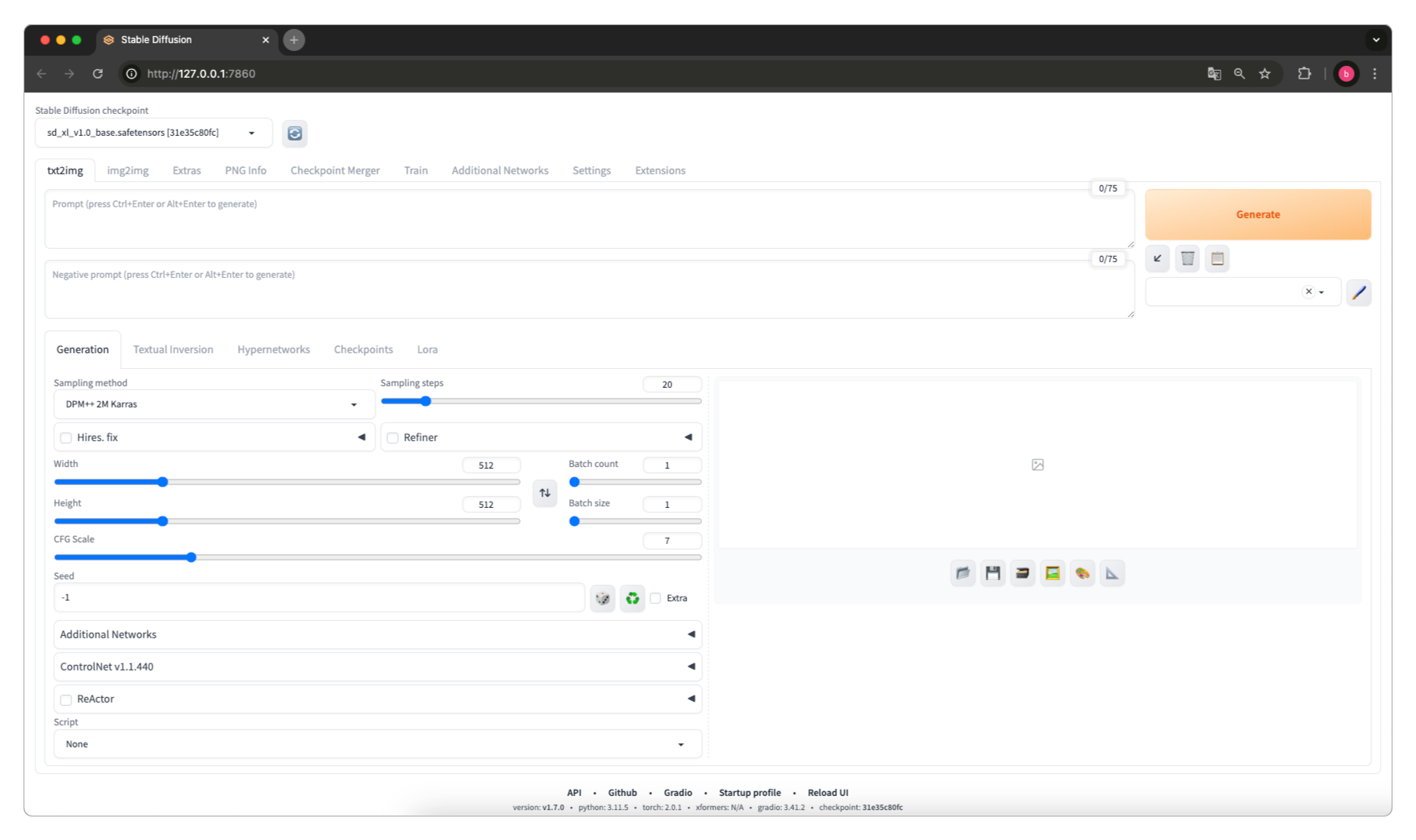
> pip install gradio
然后,就可以编写下面的代码了。
import gradio as gr
def greet(name, intensity):
return "Hello, " + name + "!" * int(intensity)
hello = gr.Interface(
fn = greet,
inputs=["text", "slider"],
outputs=["text"],
)
# 可以在launch()中添加端口号和是否在公网上显示链接
# 如果加上了share=True,那么会显示一个72小时内有效的公网链接
hello.launch(server_port=8080)
运行程序后输出结果如下。
* Running on local URL: http://127.0.0.1:8080
* Running on public URL: https://xxxxxxxxxxxxxxxxx.gradio.live
打开浏览器,界面显示如下。
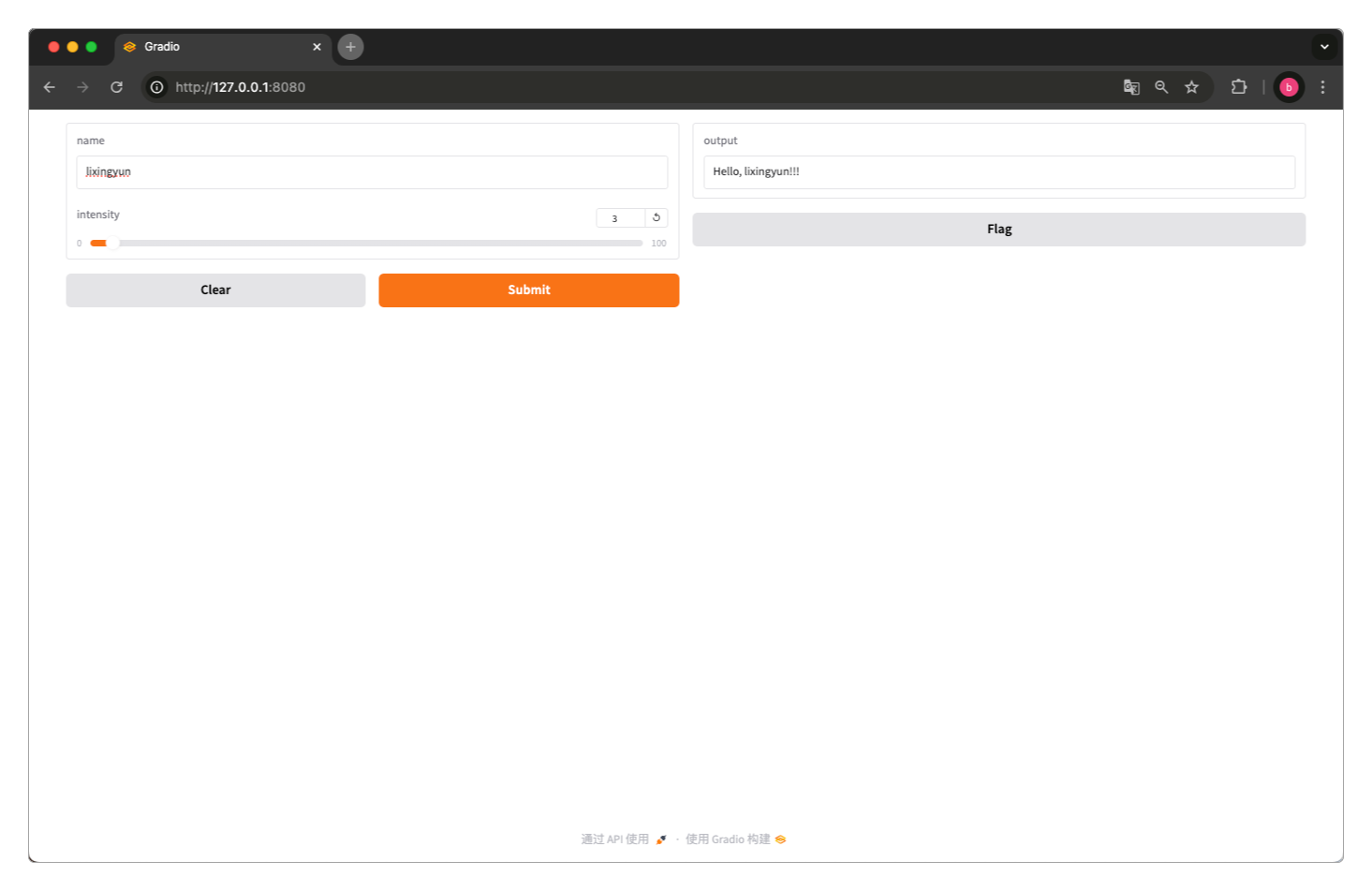
更多关于Gradio的代码如下。
import gradio as gr
import numpy as np
import matplotlib.pyplot as plt
from PIL.ImageOps import scale
def func():
pass
def button_clicked(name):
return f"Hello {name}"
# 点击事件
with gr.Blocks() as clickEvent:
text_input = gr.Textbox("点击按钮后的反馈将显示在这里")
button = gr.Button("点击我")
button.click(fn=lambda: button_clicked("test"), outputs=[text_input])
# 输出matplotlib
def output_matplotlib():
x = np.linspace(0, 10, 100)
fig, ax = plt.subplots()
ax.plot(x, np.sin(x), label='sin')
ax.plot(x, np.cos(x), label='cos')
ax.legend()
return fig
def gallery_list():
images = [
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
]
# 给每张图片加上标签
results = [(img, f"图片 {i + 1}") for i, img in enumerate(images)]
return results
'''
绘图界面
'''
image = gr.Interface(
fn=output_matplotlib,
inputs=None,
outputs=gr.Plot()
)
'''
画廊界面
'''
gallery = gr.Interface(
fn=gallery_list,
inputs=None,
outputs=gr.Gallery(label="图片列表", columns=4)
)
'''
常见组件界面
'''
normal = gr.Interface(
fn=func,
# 常用输入
inputs=[
# 常用文本输入框
gr.Text(label="账号", placeholder="请输入账号"),
gr.Text(label="密码", placeholder="请输入密码", type="password"),
gr.Text(label="邮箱", placeholder="请输入邮箱", type="email"),
gr.Textbox(label="个人信息", lines=3, max_lines=5, placeholder="请填写个人信息"),
gr.TextArea(label="个人介绍", lines=3, max_lines=5, placeholder="请输入个人介绍"),
# 常用数字输入框
gr.Number(label="年龄", precision=0),
gr.Number(label="身高(CM)", precision=2),
gr.Number(label="资产", precision=2, value=10000),
# 不可编辑
gr.Number(label="资产", precision=2, value=10000, interactive=False),
gr.Slider(label="体重", minimum=0, maximum=100, step=1),
gr.Slider(label="血型", minimum=1, maximum=4, step=1, value=3, info="1:A型,2:B型,3:O型,4:AB型"),
# 勾选框
gr.Checkbox(label="是否同意"),
# 单选框
gr.Radio(label="性别", choices=["男", "女", "保密"], type="index"),
# 多选框
gr.CheckboxGroup(label="爱好", choices=["足球", "篮球", "游泳", "跑步"]),
# 下拉框
gr.Dropdown(label="城市",
choices=["北京", "上海", "广州", "深圳", "成都", "西安", "武汉", "郑州", "重庆", "天津", "昆明",
"杭州", "南京", "珠海"]),
# 文件选择器(允许上传文件夹)
gr.File(label="上传文件", file_count="directory", file_types=None),
# 图片选择器
gr.Image(label="上传图片", width=224, height=224),
# 视频选择器
gr.Video(label="上传视频", width=224, height=224),
# 按钮
# 可以给按钮设置icon
gr.Button("点击我", icon="这里设置图标资源路径"),
gr.Button("提交", variant="primary"),
gr.Button("重置", variant="secondary"),
gr.Button("取消", variant="stop"),
gr.Button("返回", variant="huggingface"),
],
# 常用输出
outputs=[
gr.Text(label="输出", placeholder="这里显示输出内容"),
gr.Textbox(label="输出", lines=3, max_lines=5, placeholder="这里显示输出内容"),
gr.TextArea(label="输出", lines=3, max_lines=5, placeholder="这里显示输出内容"),
],
)
'''
行排版
'''
with gr.Blocks() as row:
with gr.Row():
# 通过scale参数调整组件的宽度占比
input1 = gr.Text(label="输入", placeholder="请输入内容", scale=2),
button1 = gr.Button("欢迎", variant="primary", scale=3)
text1 = gr.Text(label="输出", placeholder="显示输出内容", scale=5)
with gr.Row():
input2 = gr.Text(label="输入", placeholder="请输入内容", scale=5),
button2 = gr.Button("欢迎", variant="primary", scale=3)
text2 = gr.Text(label="输出", placeholder="显示输出内容", scale=2)
button1.click(fn=button_clicked, inputs=input1, outputs=text1)
button2.click(fn=button_clicked, inputs=input2, outputs=text2)
'''
列排版
'''
with gr.Blocks() as column:
with gr.Row():
with gr.Column(scale=5):
input11 = gr.Textbox(label="提示词", lines=2, placeholder="请输入提示词"),
input21 = gr.Textbox(label="反向提示词", lines=2, placeholder="请输入反向提示词"),
with gr.Column(scale=2):
button11 = gr.Button(">>>"),
button21 = gr.Button("保存"),
button31 = gr.Button("文件"),
button41 = gr.Button("删除"),
with gr.Column(scale=3):
button51 = gr.Button("生成", variant="primary"),
with gr.Row():
dropbox11 = gr.Dropdown(label="选择样式", choices=["样式1", "样式2", "样式3"]),
dropbox21 = gr.Dropdown(label="选择条件", choices=["条件1", "条件2", "条件3"]),
'''
标签排版
'''
with gr.Blocks() as tab:
with gr.Tab("文生图"):
with gr.Blocks() as column2:
with gr.Row():
with gr.Column(scale=5):
input12 = gr.Textbox(label="提示词", lines=2, placeholder="请输入提示词"),
input22 = gr.Textbox(label="反向提示词", lines=2, placeholder="请输入反向提示词"),
with gr.Column(scale=2):
button12 = gr.Button(">>>"),
button22 = gr.Button("保存"),
button32 = gr.Button("文件"),
button42 = gr.Button("删除"),
with gr.Column(scale=3):
button52 = gr.Button("生成", variant="primary"),
with gr.Row():
dropbox12 = gr.Dropdown(label="选择样式", choices=["样式1", "样式2", "样式3"]),
dropbox22 = gr.Dropdown(label="选择条件", choices=["条件1", "条件2", "条件3"]),
with gr.Tab("图生图"):
pass
with gr.Tab("文生语音"):
pass
with gr.Tab("文生视频"):
pass
with gr.Tab("模型训练"):
pass
with gr.Tab("设置"):
pass
'''
折叠排版
'''
with gr.Blocks() as block:
with gr.Accordion("模型参数", open=False):
gr.Textbox(label="提示词", lines=2, placeholder="请输入提示词"),
gr.Textbox(label="反向提示词", lines=2, placeholder="请输入反向提示词"),
gr.Textbox(label="步数", lines=2, placeholder="请输入步数"),
gr.Textbox(label="学习率", lines=2, placeholder="请输入学习率"),
# 启动点击事件测试
# clickEvent.launch(server_port=8080)
# 启动绘图输出测试
# image.launch(server_port=8080)
# 启动画廊输出测试
# gallery.launch(server_port=8080)
# 启动常见组件测试
# normal.launch(server_port=8080)
# 行排版测试
# row.launch(server_port=8080)
# 列排版测试
# column.launch(server_port=8080)
# 标签排版测试
# tab.launch(server_port=8080)
# 折叠排版测试
block.launch(server_port=8080)
仿Stable Diffusion界面
'''
仿Stable Diffusion界面
'''
import gradio as gr
with gr.Blocks() as stable_diffusion:
# 行布局:模型选择
with gr.Row():
gr.Dropdown(label="stable diffusion模型", choices=["stable-diffusion-v1-5", "stable-diffusion-2-1-base", "stable-diffusion-2-1"])
gr.Dropdown(label="外挂VAE模型", choices=["VAE1", "VAE2", "VAE3"]),
gr.Slider(label="CLIP终止层数", minimum=0, maximum=100, step=1, value=10)
# 标签布局
with gr.Tab("文生图"):
with gr.Row():
with gr.Column(scale=4):
prompt = gr.Textbox(label="提示词", lines=2)
negative_prompt = gr.Textbox(label="负提示词", lines=2)
with gr.Column(scale=2):
gr.Button("生成图片", variant="primary")
with gr.Row():
gr.Button("下载", variant="secondary")
gr.Button("保存", variant="secondary")
gr.Button("删除", variant="secondary")
gr.Dropdown(label="stable diffusion模型", choices=["stable-diffusion-v1-5", "stable-diffusion-2-1-base", "stable-diffusion-2-1"])
# 子标签布局
with gr.Tab("生成"):
with gr.Row():
with gr.Column():
gr.Slider(label="采样步数", minimum=1, maximum=100, step=1, value=8)
gr.Radio(label="采样方法", choices=[
"DPM++2M Karras", "DPM++ SDE Karras", "DPM++2M SDE Exponential", "DPM++2M SDE Karras", "Euler a",
"Euler", "LMS", "Heun", "DPM2", "DPM2 a", "DPM++2M", "DPM++2M SDE", "DPM++ SDE", "DPM fast",
"DPM adaptive", "LMS Karras", "DPM2 Karras", "DPM2 a Karras", "DDIM", "PLMS", "UniPC", "VLM",
"K-LMS", "K-Euler a", "K-Euler", "K-Heun", "K-DPM2", "K-DPM2 a", "K-DPM++2M", "K-DPM++2M SDE",
"K-DPM++ SDE", "K-DPM fast", "K-DPM adaptive", "K-LMS Karras", "K-DPM2 Karras", "K-DPM2 a Karras",
"K-DDIM", "K-PLMS", "K-UniPC", "K-VLM"
], type="index", value=0)
# 折叠布局
with gr.Accordion("高分辨率修复"):
with gr.Row():
gr.Dropdown(label="放大算法", choices=["SDXL", "SDXL Refiner", "SDXL VAE", "SDXL VAE Refiner"])
gr.Slider(label="高分迭代步数")
gr.Slider(label="重绘幅度")
with gr.Row():
gr.Slider(label="放大倍数")
gr.Slider(label="将宽度调整为")
gr.Slider(label="将高度调整为")
# 折叠布局
with gr.Accordion("Refiner"):
with gr.Row():
gr.Dropdown(label="模型",
choices=["SDXL", "SDXL Refiner", "SDXL VAE", "SDXL VAE Refiner"])
gr.Slider(label="切换时机")
with gr.Row():
with gr.Column(scale=2):
gr.Slider(label="宽度", minimum=1, maximum=1000, step=1, value=512)
gr.Slider(label="高度", minimum=1, maximum=1000, step=1, value=512)
with gr.Column(scale=1, min_width=1):
gr.Slider(label="总批次", minimum=1, maximum=100, step=1, value=10, min_width=1)
gr.Slider(label="单批数量", minimum=1, maximum=100, step=1, value=10, min_width=1)
gr.Slider(label="提示词引导系数", minimum=0, maximum=100, step=1, value=0)
with gr.Column():
gr.Gallery(label="图片", value=[
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
"文章封面1.png",
"文章封面2.png",
])
with gr.Row():
gr.Button("发送到重绘", variant="secondary")
gr.Button("发送到后期处理", variant="secondary")
gr.Button("下载", variant="secondary")
gr.Textbox(label="图像信息", lines=5)
with gr.Tab("嵌入式"):
pass
with gr.Tab("超网络"):
pass
with gr.Tab("模型"):
pass
with gr.Tab("LoRA"):
pass
with gr.Tab("图生图"):
pass
with gr.Tab("后期处理"):
pass
with gr.Tab("PNG图片信息"):
pass
with gr.Tab("模型融合"):
pass
with gr.Tab("训练"):
pass
with gr.Tab("无边图像浏览"):
pass
with gr.Tab("图生图"):
pass
stable_diffusion.launch(server_port=8080)
完成后的效果如下图所示。
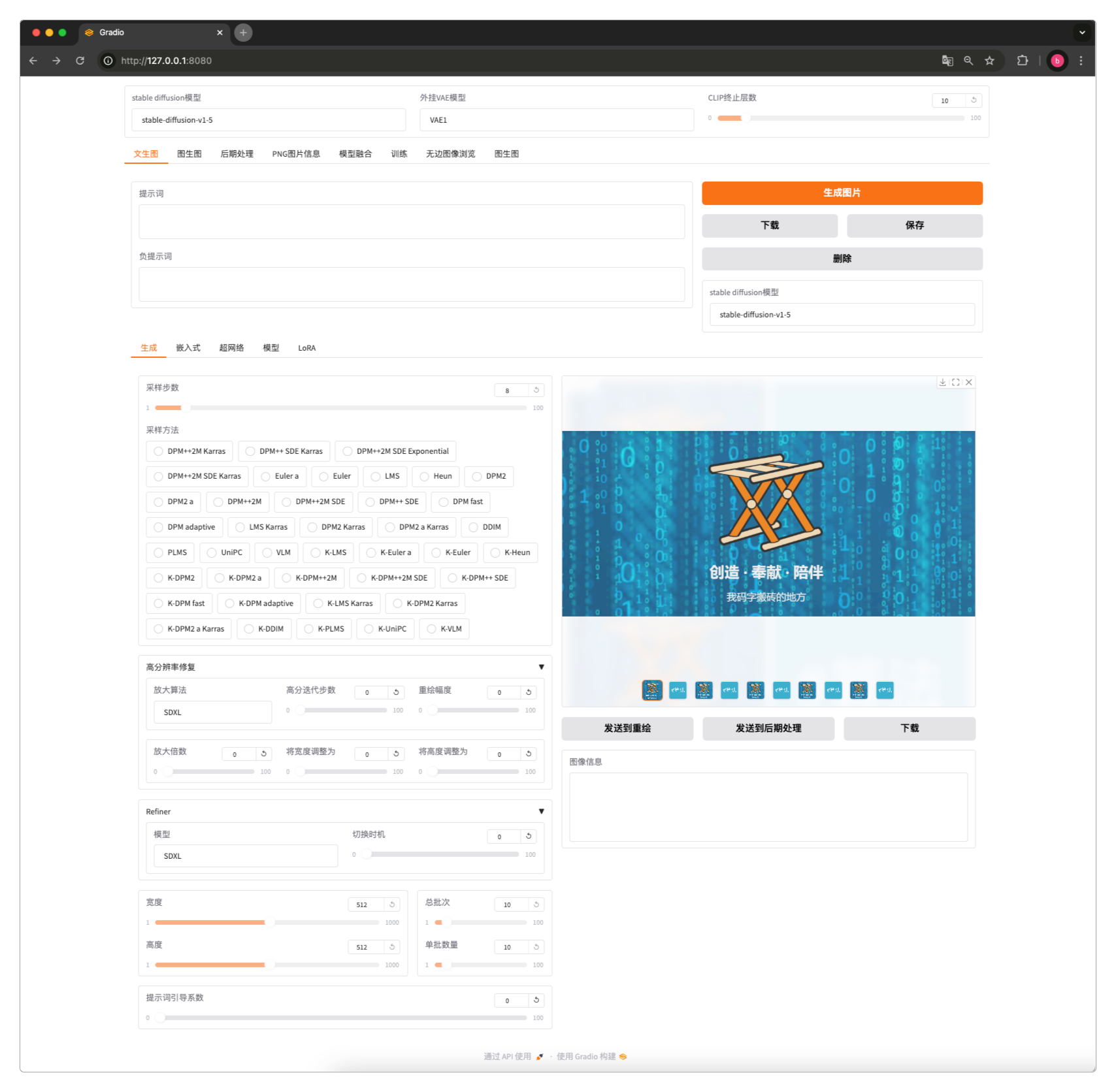
感谢支持
更多内容,请移步《超级个体》。